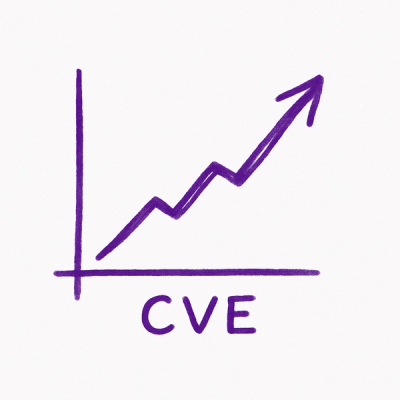
Security News
New CVE Forecasting Tool Predicts 47,000 Disclosures in 2025
CVEForecast.org uses machine learning to project a record-breaking surge in vulnerability disclosures in 2025.
Database components for QuickAPI including data models, attributes, and EF Core extensions
QuickAPI.Database
is a foundational library for database components in QuickAPI. It provides a set of base models, a core DbContext
implementation, an interface for tenant-based multi-tenancy support, and custom data annotations for EF Core models.
This package is designed to be used in backend projects that require database operations with Entity Framework Core.
BaseModel
class with common fields such as Id
, CreatedAt
, ModifiedAt
, and IsDeleted
.ITenantModel
to enforce tenant-based filtering.BaseContext
class that automatically applies query filters, tracks modifications, and handles tenant-aware queries.To install this package via NuGet:
dotnet add package QuickAPI.Database
This package requires EF Core. Ensure you have installed Microsoft.EntityFrameworkCore
in your project.
All entity models should inherit from BaseModel
to get common fields and automatic tracking.
using QuickAPI.Database.DataModels;
public class Product : BaseModel
{
public decimal Price { get; set; }
}
To support multi-tenancy, a model should implement ITenantModel
:
using QuickAPI.Database.DataModels;
public class Order : BaseModel, ITenantModel
{
public Guid TenantId { get; set; }
public decimal TotalAmount { get; set; }
}
The BaseContext
class automatically applies:
_tenantProvider
using Microsoft.EntityFrameworkCore;
using QuickAPI.Database.Data;
public class AppDbContext : BaseContext
{
public AppDbContext(DbContextOptions<AppDbContext> options, ITenantProvider tenantProvider)
: base(options, tenantProvider)
{
}
public DbSet<Product> Products { get; set; }
}
Use built-in attributes for defining SQL constraints directly in your models.
[SqlDefaultValue("getdate()")]
public DateTimeOffset CreatedAt { get; set; }
[SqlComputed("Price * Quantity", stored: true)]
public decimal TotalCost { get; set; }
[SqlPrimaryKey("PK_CustomKey", "Id")]
public class CustomEntity
{
public int Id { get; set; }
}
This project is licensed under the Apache 2.0 License.
FAQs
Database components for QuickAPI including data models, attributes, and EF Core extensions
We found that quickapi.database demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
CVEForecast.org uses machine learning to project a record-breaking surge in vulnerability disclosures in 2025.
Security News
Browserslist-rs now uses static data to reduce binary size by over 1MB, improving memory use and performance for Rust-based frontend tools.
Research
Security News
Eight new malicious Firefox extensions impersonate games, steal OAuth tokens, hijack sessions, and exploit browser permissions to spy on users.