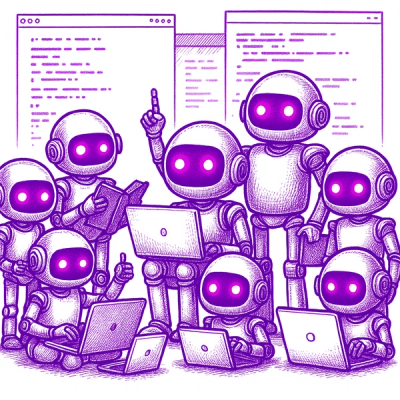
Security News
Open Source CAI Framework Handles Pen Testing Tasks up to 3,600× Faster Than Humans
CAI is a new open source AI framework that automates penetration testing tasks like scanning and exploitation up to 3,600× faster than humans.
Rapid.Microservices.Framework.Core
Advanced tools
Rapid.Microservice.Framework is a plugin-based .NET microservice framework designed for lightning-fast service development. It handles endpoint creation, database management, and job execution out-of-the-box — letting developers focus entirely on business logic.
Rapid.Microservice.Framework is a plugin-based .NET microservice framework designed for lightning-fast service development. It handles endpoint creation, database management, and job execution out-of-the-box — letting developers focus entirely on business logic.
✅ Auto-generates REST APIs from your plugins along with standard APIs
✅ Automatically creates controller classes for all your model or data model classes
⏱️ Scheduled job execution with simple XML config
💾 Automatic database handling using plugin-based storage engines
🔍 Built-in logging and monitoring for easy debugging
🔄 Plugin architecture for easy extensibility and customization
🔒 Multi-tenancy support with AppId-based data separation
💾 Built-in storage support with SQLite and other pluggable DBs
📡 RESTful querying based on AppId (multi-project/multi-tenant)
⚙️ Self-contained Windows Service for seamless deployment
🔌 Plugin-based development and logic execution (via Jobs)
🧩 Ideal for SaaS and enterprise tools
🛠️ Windows service ready-to-run executable included after build
dotnet add package Rapid.Microservice.Framework.Core
📝 Requirement You want a microservice to:
Read line coverage metrics from nightly build dumps.
Save snapshot data per project.
Expose historical coverage trends via REST APIs.
Add a reference to latest Rapid.Microservice.Framework.Core.
dotnet add package Rapid.Microservice.Framework.Core
[AutoController("CodeCoverage")]
public class CodeCoverageDataResponse : BasicResponse
{
// Inherits standard properties from BasicResponse:
// public string AppId { get; set; }
// public string Color { get; set; }
// public dynamic myData { get; set; } // User can use this field to set any kind of objects like List, Dictionary, etc.
// public string ReportDate { get; set; } // Framework automatically sets this
// public string TimeStamp { get; set; } // Framework automatically sets this
//public string ApiId
public double LineCoverage { get; set; }
public string Project { get; set; }
}
using System;
using Rapid.Microservices.Framework.Core.Common.Logger;
using Rapid.Microservices.Framework.Core.DataAccess;
using Rapid.Microservices.Framework.Core.Infrastructure.JobManager;
using Rapid.Microservices.Controller.PlugIn.Sample.ResponseModels;
namespace Rapid.Microservices.Controller.PlugIn.Sample.Jobs
{
public class CodeCoverageJob : Job<CodeCoverageDataResponse>
{
public override void DoJob(string date, IJobConfiguration jobConfiguration)
{
var AppId = jobConfiguration.AppId; // From JobConfiguration.xml
var project = jobConfiguration.Config["Project"]; // From JobConfiguration.xml
var coverageDumpPath = jobConfiguration.Config["CoverageDumpPath"]; // From JobConfiguration.xml
var lineCoverage = ReadCodeCoverageFromDump(coverageDumpPath);
try
{
var result = new CodeCoverageDataResponse
{
AppId = appId, //Id used to query this data using REST API
LineCoverage = lineCoverage,
ReportDate = date,
Project = project
};
Save(result); // Save api from framework will take of saving data with date to database
}
catch (Exception exception)
{
LoggerService.Error(this, $"Reading CodeCoverage Metrics for {appId} failed", exception);
}
}
}
private double ReadCodeCoverageFromDump(string path)
{
// Implement coverage dump parsing logic
return 78.5;
}
}
By default, the framework automatically generates controller for all DTO classes with the [AutoController] class level attribute. This allows you to access the API endpoints without creating a controller class manually.
Example:
CodeCoverageController
class automatically.In case you want to create a custom controller class to add more REST APIs, you can do so by creating a new class and inheriting from BaseController<T>
.
Create a CodeCoverageController.cs
file in your plugin project:
public class CodeCoverageController : BaseController<CodeCoverageDto>
{
// Inherits standard REST APIs:
//http(s)://<host>:port/api/codecoverage/datajson
//http(s)://<host>:port/api/codecoverage/trenddatajson
//http(s)://<host>:port/api/codecoverage/predictiondatajson
//http(s)://<host>:port/api/codecoverage/predictiontrenddatajson
// You can also add custom endpoints here if needed
}
Endpoint | Description |
---|---|
/api/CodeCoverage/DataJson/AppId | Latest snapshot data by AppId |
/api/CodeCoverage/TrendDataJson/AppId/30 | Historical trend data by AppId and duration in days |
/api/CodeCoverage/PredictionDataJson/AppId | Forecasted snapshot data (if enabled) |
/api/CodeCoverage/PredictionTrendDataJsonAppId/30 | Trend of predictions over time |
After building, your output bin folder will include:
- Rapid.Microservices.Framework.Service.exe
- configurations/
- Sample_ServiceConfiguration.xml
- Sample_JobConfiguration.xml
- install_service.bat
- uninstall_service.bat
- readme.md
- license.txt
- other binaries
Then rename Sample_ServiceConfiguration.xml to ServiceConfiguration.xml and Sample_JobConfiguration.xml to JobConfiguration.xml
Copy all files from bin to your deployment folder:
D:\Microservices\CodeCoverage\
Edit ServiceConfiguration.xml
<Configurations>
<Service>
<Id>CodeCoverage</Id>
<Name>Code Coverage Service</Name>
<DisplayName>Code Coverage Service</DisplayName>
<Description>Provides snapshot and trend of line coverage for projects</Description>
<Host>localhost</Host>
<Protocol>http</Protocol>
<Port>8010</Port>
<DbPlugIn>sqlite</DbPlugIn>
<PlugIns>CodeCoverage.PlugIn.dll</PlugIns>
<LogFilePath>D:\Microservices\CodeCoverage\logs</LogFilePath>
</Service>
<Jobs>
<Job>
<Name>CodeCoverageJob</Name>
<Enabled>True</Enabled>
<Interval>180</Interval>
</Job>
</Jobs>
<Notification>
<EmailIds>
<To>support@@vedicaai.com</To>
<Cc>operator@@vedicaai.com</Cc>
</EmailIds>
</Notification>
</Configurations>
Then in ServiceConfiguration.xml
📝 Update <PlugIns
> to your dll name.
🕒 <Interval
> is in minutes (e.g., 180 = every 3 hours).
Edit JobConfiguration.xml
Copy
Edit
<Configurations>
<Jobs>
<Job>
<Name>CodeCoverageJob</Name>
<AppId>DataElement-123</AppId>
<Project>MyProjectName</AppId>
<CoverageDumpPath>C:\Build\Artifacts\Coverage\</CoverageDumpPath>
</Job>
</Jobs>
</Configurations>
🆔 Set a unique for each Data Element.
Run from deployment folder:
install_service.bat
Then open Services (services.msc), locate Code Coverage Service, and start it.
GET http://localhost:8010/api/CodeCoverage/DataJson/DataElement-123
Here, DataElement-123
is the AppId and is case sensitive.
✅ Example Response => Latest Data Element
{
"AppId": "DataElement-123",
"LineCoverage": 78.5,
"ReportDate": "2025-04-04",
"timestamp": "2025-04-04T22:00:00Z",
"Project": "MyProjectName"
}
GET http://localhost:8010/api/CodeCoverage/TrendDataJson/myproject-123/4
Here, DataElement-123
is the AppId and 4
is the number of days data to fetch.
✅ Example Response => List of Data Elements
[
{
"AppId": "DataElement-123",
"LineCoverage": 78.5,
"ReportDate": "2025-04-04",
"timestamp": "2025-04-04T22:00:00Z",
"Project": "MyProjectName"
},
{
"AppId": "DataElement-123",
"LineCoverage": 77.5,
"ReportDate": "2025-04-03",
"timestamp": "2025-04-03T22:00:00Z",
"Project": "MyProjectName"
},
{
"AppId": "DataElement-123",
"LineCoverage": 74.5,
"ReportDate": "2025-04-02",
"timestamp": "2025-04-02T22:00:00Z",
"Project": "MyProjectName"
},
{
"AppId": "DataElement-123",
"LineCoverage": 72.5,
"ReportDate": "2025-04-01",
"timestamp": "2025-04-01T22:00:00Z",
"Project": "MyProjectName"
}
]
AppId uniquely identifies each Data Element
All saved Data Element is retrieved using AppId
REST APIs return only Data Element for the specified AppId
Jobs run at intervals configured in ServiceConfiguration.xml
Each job runs in its own execution context
Additional job classes can be added as needed and configured separately in JobConfiguration.xml
Metrics collection services
Build/test/deployment tracking
Health check monitors
Custom backend APIs for dashboards
Internal microservices for enterprise systems
Scheduled microservices (ETL, metrics, data scrapers)
Internal tools (build monitors, test metrics, deployment logs)
SaaS dashboards with project-specific APIs
Multi-Tenant Design: AppId enables scoped data separation per data element/project.
Logging: Log path configurable in ServiceConfiguration.xml.
Database: Use sqlite, postgresql, mssql via plugin-based backends.
Job Scheduling: Supports any number of jobs with independent schedules.
Plugin Architecture: Easily extend functionality by creating new plugins.
The Core Framework is not open-source, but is freely distributed via NuGet. This software is a personal passion project, developed and shared freely for the benefit of the community. It is intended to support learning, experimentation, research, in-house business applications and personal use in non-commercial settings.
This framework is not open source, but it is freely distributed via NuGet. If you encounter issues, bugs, or have suggestions, feel free to reach out to the maintainers or submit feedback via the appropriate support channels.
Item | Value |
---|---|
Package Name | Rapid.Microservice.Framework.Core |
Service Entry Point | Rapid.Microservices.Framework.Service.exe |
Config Directory | bin/configuration/ |
Endpoint Format - Snapshot data | /api/controller/datajson/appid |
Endpoint Format - Trend data | /api/controller/trenddatajson/appid/duration |
Install Command | install_service.bat |
Uninstall Command | uninstall_service.bat |
FAQs
Rapid.Microservice.Framework is a plugin-based .NET microservice framework designed for lightning-fast service development. It handles endpoint creation, database management, and job execution out-of-the-box — letting developers focus entirely on business logic.
We found that rapid.microservices.framework.core demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
CAI is a new open source AI framework that automates penetration testing tasks like scanning and exploitation up to 3,600× faster than humans.
Security News
Deno 2.4 brings back bundling, improves dependency updates and telemetry, and makes the runtime more practical for real-world JavaScript projects.
Security News
CVEForecast.org uses machine learning to project a record-breaking surge in vulnerability disclosures in 2025.