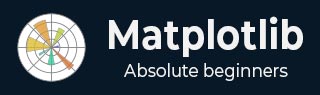
- Matplotlib - Home
- Matplotlib - Introduction
- Matplotlib - Vs Seaborn
- Matplotlib - Environment Setup
- Matplotlib - Anaconda distribution
- Matplotlib - Jupyter Notebook
- Matplotlib - Pyplot API
- Matplotlib - Simple Plot
- Matplotlib - Saving Figures
- Matplotlib - Markers
- Matplotlib - Figures
- Matplotlib - Styles
- Matplotlib - Legends
- Matplotlib - Colors
- Matplotlib - Colormaps
- Matplotlib - Colormap Normalization
- Matplotlib - Choosing Colormaps
- Matplotlib - Colorbars
- Matplotlib - Working With Text
- Matplotlib - Text properties
- Matplotlib - Subplot Titles
- Matplotlib - Images
- Matplotlib - Image Masking
- Matplotlib - Annotations
- Matplotlib - Arrows
- Matplotlib - Fonts
- Matplotlib - Font Indexing
- Matplotlib - Font Properties
- Matplotlib - Scales
- Matplotlib - LaTeX
- Matplotlib - LaTeX Text Formatting in Annotations
- Matplotlib - PostScript
- Matplotlib - Mathematical Expressions
- Matplotlib - Animations
- Matplotlib - Celluloid Library
- Matplotlib - Blitting
- Matplotlib - Toolkits
- Matplotlib - Artists
- Matplotlib - Styling with Cycler
- Matplotlib - Paths
- Matplotlib - Path Effects
- Matplotlib - Transforms
- Matplotlib - Ticks and Tick Labels
- Matplotlib - Radian Ticks
- Matplotlib - Dateticks
- Matplotlib - Tick Formatters
- Matplotlib - Tick Locators
- Matplotlib - Basic Units
- Matplotlib - Autoscaling
- Matplotlib - Reverse Axes
- Matplotlib - Logarithmic Axes
- Matplotlib - Symlog
- Matplotlib - Unit Handling
- Matplotlib - Ellipse with Units
- Matplotlib - Spines
- Matplotlib - Axis Ranges
- Matplotlib - Axis Scales
- Matplotlib - Axis Ticks
- Matplotlib - Formatting Axes
- Matplotlib - Axes Class
- Matplotlib - Twin Axes
- Matplotlib - Figure Class
- Matplotlib - Multiplots
- Matplotlib - Grids
- Matplotlib - Object-oriented Interface
- Matplotlib - PyLab module
- Matplotlib - Subplots() Function
- Matplotlib - Subplot2grid() Function
- Matplotlib - Anchored Artists
- Matplotlib - Manual Contour
- Matplotlib - Coords Report
- Matplotlib - AGG filter
- Matplotlib - Ribbon Box
- Matplotlib - Fill Spiral
- Matplotlib - Findobj Demo
- Matplotlib - Hyperlinks
- Matplotlib - Image Thumbnail
- Matplotlib - Plotting with Keywords
- Matplotlib - Create Logo
- Matplotlib - Multipage PDF
- Matplotlib - Multiprocessing
- Matplotlib - Print Stdout
- Matplotlib - Compound Path
- Matplotlib - Sankey Class
- Matplotlib - MRI with EEG
- Matplotlib - Stylesheets
- Matplotlib - Background Colors
- Matplotlib - Basemap
- Matplotlib - Event Handling
- Matplotlib - Close Event
- Matplotlib - Mouse Move
- Matplotlib - Click Events
- Matplotlib - Scroll Event
- Matplotlib - Keypress Event
- Matplotlib - Pick Event
- Matplotlib - Looking Glass
- Matplotlib - Path Editor
- Matplotlib - Poly Editor
- Matplotlib - Timers
- Matplotlib - Viewlims
- Matplotlib - Zoom Window
- Matplotlib Widgets
- Matplotlib - Cursor Widget
- Matplotlib - Annotated Cursor
- Matplotlib - Buttons Widget
- Matplotlib - Check Buttons
- Matplotlib - Lasso Selector
- Matplotlib - Menu Widget
- Matplotlib - Mouse Cursor
- Matplotlib - Multicursor
- Matplotlib - Polygon Selector
- Matplotlib - Radio Buttons
- Matplotlib - RangeSlider
- Matplotlib - Rectangle Selector
- Matplotlib - Ellipse Selector
- Matplotlib - Slider Widget
- Matplotlib - Span Selector
- Matplotlib - Textbox
- Matplotlib Plotting
- Matplotlib - Line Plots
- Matplotlib - Area Plots
- Matplotlib - Bar Graphs
- Matplotlib - Histogram
- Matplotlib - Pie Chart
- Matplotlib - Scatter Plot
- Matplotlib - Box Plot
- Matplotlib - Arrow Demo
- Matplotlib - Fancy Boxes
- Matplotlib - Zorder Demo
- Matplotlib - Hatch Demo
- Matplotlib - Mmh Donuts
- Matplotlib - Ellipse Demo
- Matplotlib - Bezier Curve
- Matplotlib - Bubble Plots
- Matplotlib - Stacked Plots
- Matplotlib - Table Charts
- Matplotlib - Polar Charts
- Matplotlib - Hexagonal bin Plots
- Matplotlib - Violin Plot
- Matplotlib - Event Plot
- Matplotlib - Heatmap
- Matplotlib - Stairs Plots
- Matplotlib - Errorbar
- Matplotlib - Hinton Diagram
- Matplotlib - Contour Plot
- Matplotlib - Wireframe Plots
- Matplotlib - Surface Plots
- Matplotlib - Triangulations
- Matplotlib - Stream plot
- Matplotlib - Ishikawa Diagram
- Matplotlib - 3D Plotting
- Matplotlib - 3D Lines
- Matplotlib - 3D Scatter Plots
- Matplotlib - 3D Contour Plot
- Matplotlib - 3D Bar Plots
- Matplotlib - 3D Wireframe Plot
- Matplotlib - 3D Surface Plot
- Matplotlib - 3D Vignettes
- Matplotlib - 3D Volumes
- Matplotlib - 3D Voxels
- Matplotlib - Time Plots and Signals
- Matplotlib - Filled Plots
- Matplotlib - Step Plots
- Matplotlib - XKCD Style
- Matplotlib - Quiver Plot
- Matplotlib - Stem Plots
- Matplotlib - Visualizing Vectors
- Matplotlib - Audio Visualization
- Matplotlib - Audio Processing
- Matplotlib Useful Resources
- Matplotlib - Quick Guide
- Matplotlib - Cheatsheet
- Matplotlib - Useful Resources
- Matplotlib - Discussion
Matplotlib - Celluloid Library
The celluloid library is a third-party library that works with Matplotlib to create animations. It simplifies the process of creating moving visuals by managing the figure and axis changes for each animation frame. It provides a user-friendly interface, allowing you to focus on the content of each frame rather than dealing with complex animation details.
Matplotlib Celluloid Library
Matplotlib is a useful Python library for creating various visualizations, such as charts and graphs. If you want to add animation to your plots, you can use the celluloid library. It simplifies the process of generating dynamic and interesting animations using Matplotlib. In this tutorial, we will go through the basics of using Matplotlib with the celluloid library to make animated plots.
Before starting, make sure you have installed the required libraries −
pip install matplotlib pip install celluloid
Creating Matplotlib Animations
Once you have installed the required libraries, let us go through the steps involved in creating Matplotlib animations using celluloid −
Step 1 − Import Libraries
Let us start by importing the necessary libraries i.e. Matplotlib for plotting, NumPy for numerical operations, and the Camera class from the celluloid library.
import matplotlib.pyplot as plt import numpy as np from celluloid import Camera
Step 2 − Setting Up Matplotlib Figure and Axis
Next, set up a standard Matplotlib figure and axis, just like you would for a static plot. These will be used to visualize the data in each frame.
fig, ax = plt.subplots()
Step 3 − Initializing the Camera Object
Now, we initialize the "Camera" object from the "celluloid" library. This object will capture each frame for the animation, allowing you to flawlessly create the final animated plot.
camera = Camera(fig)
Step 4 − Update Frames in a Loop
Inside a loop, update the plot for each frame by modifying the data or layout. In this example, the sine wave is shifted horizontally for each frame. The "camera.snap()" method captures the current frame.
num_frames = 50 for i in range(num_frames): # Update your plot for each frame x = np.linspace(0, 2 * np.pi, 100) y = np.sin(x + i * 0.1) ax.plot(x, y) # Capture the current frame camera.snap()
Step 5 − Show Animation
After capturing all frames, use the "animate()" method of the "Camera" object to create the animation and display it.
animation = camera.animate() plt.show()
Complete Example
Following is the complete example of a simple animated plot. Here, we use the celluloid library in Matplotlib to generate an animated plot of a sine wave. The animation iterates through a specified number of frames (num_frames), updating the sine wave by shifting it horizontally in each frame −
import matplotlib.pyplot as plt import numpy as np from celluloid import Camera # Setting up Matplotlib figure and axis fig, ax = plt.subplots() camera = Camera(fig) # Creating animated plot num_frames = 50 for i in range(num_frames): x = np.linspace(0, 2 * np.pi, 100) y = np.sin(x + i * 0.1) # Updating plot for each frame ax.plot(x, y) # Capturing the frame camera.snap() # Displaying the animation animation = camera.animate() plt.show()
The resulting animation shows the sine wave oscillating horizontally, creating a visual representation of a dynamic signal. The celluloid library captures each frame, allowing the animation to be displayed
Animated Scatter Plot
An animated scatter plot is a dynamic visualization that shows points moving or changing over time. Each point on the plot represents data, and as time progresses, the positions of these points changes, creating a visually engaging animation.
Example
In here, we are generating an animated scatter plot using the celluloid library in Matplotlib. The animation iterates through a specified number of frames (num_frames) and updates the scatter plot by generating random x and y coordinates for each frame −
import matplotlib.pyplot as plt import numpy as np from celluloid import Camera # Setting up Matplotlib figure and axis fig, ax = plt.subplots() camera = Camera(fig) # Creating animated scatter plot num_frames = 50 for i in range(num_frames): x = np.random.rand(20) y = np.random.rand(20) # Updating scatter plot for each frame ax.scatter(x, y) # Capturing the frame camera.snap() # Displaying the animated scatter plot animation = camera.animate() plt.show()
The resulting animation shows a dynamic set of points moving randomly within the plot. The celluloid library captures each frame, allowing the animated scatter plot to be displayed.
Animated Bar Chart
In an animated bar chart, bars representing different categories fluctuates in height as the animation progresses, creating a visual of how values in each category change over time.
Example
In this example, we are creating an animated bar chart with random bar heights in each frame −
import matplotlib.pyplot as plt import numpy as np from celluloid import Camera # Setting up Matplotlib figure and axis fig, ax = plt.subplots() camera = Camera(fig) # Creating animated bar chart num_frames = 50 x = np.arange(10) for i in range(num_frames): y = np.random.randint(1, 10, size=10) # Updating bar chart for each frame ax.bar(x, y) # Capturing the frame camera.snap() # Displaying the animated bar chart animation = camera.animate() plt.show()