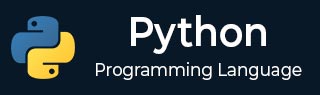
- Python - Home
- Python - Overview
- Python - History
- Python - Features
- Python vs C++
- Python - Hello World Program
- Python - Application Areas
- Python - Interpreter
- Python - Environment Setup
- Python - Virtual Environment
- Python - Basic Syntax
- Python - Variables
- Python - Data Types
- Python - Type Casting
- Python - Unicode System
- Python - Literals
- Python - Operators
- Python - Arithmetic Operators
- Python - Comparison Operators
- Python - Assignment Operators
- Python - Logical Operators
- Python - Bitwise Operators
- Python - Membership Operators
- Python - Identity Operators
- Python - Operator Precedence
- Python - Comments
- Python - User Input
- Python - Numbers
- Python - Booleans
- Python - Control Flow
- Python - Decision Making
- Python - If Statement
- Python - If else
- Python - Nested If
- Python - Match-Case Statement
- Python - Loops
- Python - for Loops
- Python - for-else Loops
- Python - While Loops
- Python - break Statement
- Python - continue Statement
- Python - pass Statement
- Python - Nested Loops
- Python Functions & Modules
- Python - Functions
- Python - Default Arguments
- Python - Keyword Arguments
- Python - Keyword-Only Arguments
- Python - Positional Arguments
- Python - Positional-Only Arguments
- Python - Arbitrary Arguments
- Python - Variables Scope
- Python - Function Annotations
- Python - Modules
- Python - Built in Functions
- Python Strings
- Python - Strings
- Python - Slicing Strings
- Python - Modify Strings
- Python - String Concatenation
- Python - String Formatting
- Python - Escape Characters
- Python - String Methods
- Python - String Exercises
- Python Lists
- Python - Lists
- Python - Access List Items
- Python - Change List Items
- Python - Add List Items
- Python - Remove List Items
- Python - Loop Lists
- Python - List Comprehension
- Python - Sort Lists
- Python - Copy Lists
- Python - Join Lists
- Python - List Methods
- Python - List Exercises
- Python Tuples
- Python - Tuples
- Python - Access Tuple Items
- Python - Update Tuples
- Python - Unpack Tuples
- Python - Loop Tuples
- Python - Join Tuples
- Python - Tuple Methods
- Python - Tuple Exercises
- Python Sets
- Python - Sets
- Python - Access Set Items
- Python - Add Set Items
- Python - Remove Set Items
- Python - Loop Sets
- Python - Join Sets
- Python - Copy Sets
- Python - Set Operators
- Python - Set Methods
- Python - Set Exercises
- Python Dictionaries
- Python - Dictionaries
- Python - Access Dictionary Items
- Python - Change Dictionary Items
- Python - Add Dictionary Items
- Python - Remove Dictionary Items
- Python - Dictionary View Objects
- Python - Loop Dictionaries
- Python - Copy Dictionaries
- Python - Nested Dictionaries
- Python - Dictionary Methods
- Python - Dictionary Exercises
- Python Arrays
- Python - Arrays
- Python - Access Array Items
- Python - Add Array Items
- Python - Remove Array Items
- Python - Loop Arrays
- Python - Copy Arrays
- Python - Reverse Arrays
- Python - Sort Arrays
- Python - Join Arrays
- Python - Array Methods
- Python - Array Exercises
- Python File Handling
- Python - File Handling
- Python - Write to File
- Python - Read Files
- Python - Renaming and Deleting Files
- Python - Directories
- Python - File Methods
- Python - OS File/Directory Methods
- Python - OS Path Methods
- Object Oriented Programming
- Python - OOPs Concepts
- Python - Classes & Objects
- Python - Class Attributes
- Python - Class Methods
- Python - Static Methods
- Python - Constructors
- Python - Access Modifiers
- Python - Inheritance
- Python - Polymorphism
- Python - Method Overriding
- Python - Method Overloading
- Python - Dynamic Binding
- Python - Dynamic Typing
- Python - Abstraction
- Python - Encapsulation
- Python - Interfaces
- Python - Packages
- Python - Inner Classes
- Python - Anonymous Class and Objects
- Python - Singleton Class
- Python - Wrapper Classes
- Python - Enums
- Python - Reflection
- Python Errors & Exceptions
- Python - Syntax Errors
- Python - Exceptions
- Python - try-except Block
- Python - try-finally Block
- Python - Raising Exceptions
- Python - Exception Chaining
- Python - Nested try Block
- Python - User-defined Exception
- Python - Logging
- Python - Assertions
- Python - Built-in Exceptions
- Python Multithreading
- Python - Multithreading
- Python - Thread Life Cycle
- Python - Creating a Thread
- Python - Starting a Thread
- Python - Joining Threads
- Python - Naming Thread
- Python - Thread Scheduling
- Python - Thread Pools
- Python - Main Thread
- Python - Thread Priority
- Python - Daemon Threads
- Python - Synchronizing Threads
- Python Synchronization
- Python - Inter-thread Communication
- Python - Thread Deadlock
- Python - Interrupting a Thread
- Python Networking
- Python - Networking
- Python - Socket Programming
- Python - URL Processing
- Python - Generics
- Python Libraries
- NumPy Tutorial
- Pandas Tutorial
- SciPy Tutorial
- Matplotlib Tutorial
- Django Tutorial
- OpenCV Tutorial
- Python Miscellenous
- Python - Date & Time
- Python - Maths
- Python - Iterators
- Python - Generators
- Python - Closures
- Python - Decorators
- Python - Recursion
- Python - Reg Expressions
- Python - PIP
- Python - Database Access
- Python - Weak References
- Python - Serialization
- Python - Templating
- Python - Output Formatting
- Python - Performance Measurement
- Python - Data Compression
- Python - CGI Programming
- Python - XML Processing
- Python - GUI Programming
- Python - Command-Line Arguments
- Python - Docstrings
- Python - JSON
- Python - Sending Email
- Python - Further Extensions
- Python - Tools/Utilities
- Python - GUIs
- Python Advanced Concepts
- Python - Abstract Base Classes
- Python - Custom Exceptions
- Python - Higher Order Functions
- Python - Object Internals
- Python - Memory Management
- Python - Metaclasses
- Python - Metaprogramming with Metaclasses
- Python - Mocking and Stubbing
- Python - Monkey Patching
- Python - Signal Handling
- Python - Type Hints
- Python - Automation Tutorial
- Python - Humanize Package
- Python - Context Managers
- Python - Coroutines
- Python - Descriptors
- Python - Diagnosing and Fixing Memory Leaks
- Python - Immutable Data Structures
- Python Useful Resources
- Python - Questions & Answers
- Python - Interview Questions & Answers
- Python - Online Quiz
- Python - Quick Guide
- Python - Reference
- Python - Cheatsheet
- Python - Projects
- Python - Useful Resources
- Python - Discussion
- Python Compiler
- NumPy Compiler
- Matplotlib Compiler
- SciPy Compiler
Python - Diagnosing and Fixing Memory Leaks
Memory leaks occur when a program incorrectly manages memory allocations which resulting in reduced available memory and potentially causing the program to slow down or crash.
In Python, memory management is generally handled by the interpreter but memory leaks can still happen especially in long-running applications. Diagnosing and fixing memory leaks in Python involves understanding how memory is allocated, identifying problematic areas and applying appropriate solutions.
Causes of Memory Leaks in Python
Memory leaks in Python can arise from several causes, primarily revolving around how objects are referenced and managed. Here are some common causes of memory leaks in Python −
1. Unreleased References
When objects are no longer needed but still referenced somewhere in the code then they are not de-allocated which leads to memory leaks. Here is the example of it −
def create_list(): my_list = [1] * (10**6) return my_list my_list = create_list() # If my_list is not cleared or reassigned, it continues to consume memory. print(my_list)
Output
[1, 1, 1, 1, ............ ............ 1, 1, 1, 1]
2. Circular References
Circular references in Python can lead to memory leaks if not managed properly but Python's cyclic garbage collector can handle many cases automatically.
For understanding how to detect and break circular references we can use the tools such as the gc and weakref modules. These tools are crucial for efficient memory management in complex Python applications. Following is the example of circular references −
class Node: def __init__(self, value): self.value = value self.next = None a = Node(1) b = Node(2) a.next = b b.next = a # 'a' and 'b' reference each other, creating a circular reference.
3. Global Variables
Variables declared at the global scope persist for the lifetime of the program which potentially causing memory leaks if not managed properly. Below is the example of it −
large_data = [1] * (10**6) def process_data(): global large_data # Use large_data pass # large_data remains in memory as long as the program runs.
4. Long-Lived Objects
Objects that persist for the lifetime of the application can cause memory issues if they accumulate over time. Here is the example −
cache = {} def cache_data(key, value): cache[key] = value # Cached data remains in memory until explicitly cleared.
5. Improper Use of Closures
Closures that capture and retain references to large objects can inadvertently cause memory leaks. Below is the example of it −
def create_closure(): large_object = [1] * (10**6) def closure(): return large_object return closure my_closure = create_closure() # The large_object is retained by the closure, causing a memory leak.
Tools for Diagnosing Memory Leaks
Diagnosing memory leaks in Python can be challenging but there are several tools and techniques available to help identify and resolve these issues. Here are some of the most effective tools and methods for diagnosing memory leaks in Python −
1. Using the "gc" Module
The gc module can help in identifying objects that are not being collected by the garbage collector. Following is the example of diagnosing the memory leaks using the gc module −
import gc # Enable automatic garbage collection gc.enable() # Collect garbage and return unreachable objects unreachable_objects = gc.collect() print(f"Unreachable objects: {unreachable_objects}") # Get a list of all objects tracked by the garbage collector all_objects = gc.get_objects() print(f"Number of tracked objects: {len(all_objects)}")
Output
Unreachable objects: 51 Number of tracked objects: 6117
2. Using "tracemalloc"
The tracemalloc module is used to trace memory allocations in Python. It is helpful for tracking memory usage and identifying where memory is being allocated. Following is the example of diagnosing the memory leaks using the tracemalloc module −
import tracemalloc # Start tracing memory allocations tracemalloc.start() # our code here a = 10 b = 20 c = a+b # Take a snapshot of current memory usage snapshot = tracemalloc.take_snapshot() # Display the top 10 memory-consuming lines top_stats = snapshot.statistics('lineno') for stat in top_stats[:10]: print(stat)
Output
C:\Users\Niharikaa\Desktop\sample.py:7: size=400 B, count=1, average=400 B
3. Using "memory_profiler"
The memory_profiler is a module for monitoring memory usage of a Python program. It provides a decorator to profile functions and a command-line tool for line-by-line memory usage analysis. In the below example we are diagnosing the memory leaks using the memory_profiler module −
from memory_profiler import profile @profile def my_function(): # our code here a = 10 b = 20 c = a+b if __name__ == "__main__": my_function()
Output
Line # Mem usage Increment Occurrences Line ====================================================================== 3 49.1 MiB 49.1 MiB 1 @profile 4 def my_function(): 5 # Your code here 6 49.1 MiB 0.0 MiB 1 a = 10 7 49.1 MiB 0.0 MiB 1 b = 20 8 49.1 MiB 0.0 MiB 1 c = a+b
Fixing Memory Leaks
Once a memory leak is identified we can fix the memory leaks,, which involves locating and eliminating unnecessary references to objects.
- Eliminate Global Variables: Avoid using global variables unless and untill absolutely necessary. Instead we can use local variables or pass objects as arguments to functions.
- Break Circular References: Use weak references to break cycles where possible. The weakref module allows us to create weak references that do not prevent garbage collection.
- Manual Cleanup: Explicitly delete objects or remove references when they are no longer needed.
- Use Context Managers: Ensure resources that are properly cleaned up using context managers i.e. with statement.
- Optimize Data Structures Use appropriate data structures that do not unnecessarily hold onto references.
Finally we can conclude Diagnosing and fixing memory leaks in Python involves identifying lingering references by using tools like gc, memory_profiler and tracemalloc etc to track memory usage and implementing fixes such as removing unnecessary references and breaking circular references.
By following these steps, we can ensure our Python programs use memory efficiently and avoid memory leaks.