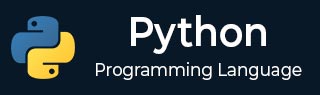
- Python - Home
- Python - Overview
- Python - History
- Python - Features
- Python vs C++
- Python - Hello World Program
- Python - Application Areas
- Python - Interpreter
- Python - Environment Setup
- Python - Virtual Environment
- Python - Basic Syntax
- Python - Variables
- Python - Data Types
- Python - Type Casting
- Python - Unicode System
- Python - Literals
- Python - Operators
- Python - Arithmetic Operators
- Python - Comparison Operators
- Python - Assignment Operators
- Python - Logical Operators
- Python - Bitwise Operators
- Python - Membership Operators
- Python - Identity Operators
- Python - Operator Precedence
- Python - Comments
- Python - User Input
- Python - Numbers
- Python - Booleans
- Python - Control Flow
- Python - Decision Making
- Python - If Statement
- Python - If else
- Python - Nested If
- Python - Match-Case Statement
- Python - Loops
- Python - for Loops
- Python - for-else Loops
- Python - While Loops
- Python - break Statement
- Python - continue Statement
- Python - pass Statement
- Python - Nested Loops
- Python Functions & Modules
- Python - Functions
- Python - Default Arguments
- Python - Keyword Arguments
- Python - Keyword-Only Arguments
- Python - Positional Arguments
- Python - Positional-Only Arguments
- Python - Arbitrary Arguments
- Python - Variables Scope
- Python - Function Annotations
- Python - Modules
- Python - Built in Functions
- Python Strings
- Python - Strings
- Python - Slicing Strings
- Python - Modify Strings
- Python - String Concatenation
- Python - String Formatting
- Python - Escape Characters
- Python - String Methods
- Python - String Exercises
- Python Lists
- Python - Lists
- Python - Access List Items
- Python - Change List Items
- Python - Add List Items
- Python - Remove List Items
- Python - Loop Lists
- Python - List Comprehension
- Python - Sort Lists
- Python - Copy Lists
- Python - Join Lists
- Python - List Methods
- Python - List Exercises
- Python Tuples
- Python - Tuples
- Python - Access Tuple Items
- Python - Update Tuples
- Python - Unpack Tuples
- Python - Loop Tuples
- Python - Join Tuples
- Python - Tuple Methods
- Python - Tuple Exercises
- Python Sets
- Python - Sets
- Python - Access Set Items
- Python - Add Set Items
- Python - Remove Set Items
- Python - Loop Sets
- Python - Join Sets
- Python - Copy Sets
- Python - Set Operators
- Python - Set Methods
- Python - Set Exercises
- Python Dictionaries
- Python - Dictionaries
- Python - Access Dictionary Items
- Python - Change Dictionary Items
- Python - Add Dictionary Items
- Python - Remove Dictionary Items
- Python - Dictionary View Objects
- Python - Loop Dictionaries
- Python - Copy Dictionaries
- Python - Nested Dictionaries
- Python - Dictionary Methods
- Python - Dictionary Exercises
- Python Arrays
- Python - Arrays
- Python - Access Array Items
- Python - Add Array Items
- Python - Remove Array Items
- Python - Loop Arrays
- Python - Copy Arrays
- Python - Reverse Arrays
- Python - Sort Arrays
- Python - Join Arrays
- Python - Array Methods
- Python - Array Exercises
- Python File Handling
- Python - File Handling
- Python - Write to File
- Python - Read Files
- Python - Renaming and Deleting Files
- Python - Directories
- Python - File Methods
- Python - OS File/Directory Methods
- Python - OS Path Methods
- Object Oriented Programming
- Python - OOPs Concepts
- Python - Classes & Objects
- Python - Class Attributes
- Python - Class Methods
- Python - Static Methods
- Python - Constructors
- Python - Access Modifiers
- Python - Inheritance
- Python - Polymorphism
- Python - Method Overriding
- Python - Method Overloading
- Python - Dynamic Binding
- Python - Dynamic Typing
- Python - Abstraction
- Python - Encapsulation
- Python - Interfaces
- Python - Packages
- Python - Inner Classes
- Python - Anonymous Class and Objects
- Python - Singleton Class
- Python - Wrapper Classes
- Python - Enums
- Python - Reflection
- Python Errors & Exceptions
- Python - Syntax Errors
- Python - Exceptions
- Python - try-except Block
- Python - try-finally Block
- Python - Raising Exceptions
- Python - Exception Chaining
- Python - Nested try Block
- Python - User-defined Exception
- Python - Logging
- Python - Assertions
- Python - Built-in Exceptions
- Python Multithreading
- Python - Multithreading
- Python - Thread Life Cycle
- Python - Creating a Thread
- Python - Starting a Thread
- Python - Joining Threads
- Python - Naming Thread
- Python - Thread Scheduling
- Python - Thread Pools
- Python - Main Thread
- Python - Thread Priority
- Python - Daemon Threads
- Python - Synchronizing Threads
- Python Synchronization
- Python - Inter-thread Communication
- Python - Thread Deadlock
- Python - Interrupting a Thread
- Python Networking
- Python - Networking
- Python - Socket Programming
- Python - URL Processing
- Python - Generics
- Python Libraries
- NumPy Tutorial
- Pandas Tutorial
- SciPy Tutorial
- Matplotlib Tutorial
- Django Tutorial
- OpenCV Tutorial
- Python Miscellenous
- Python - Date & Time
- Python - Maths
- Python - Iterators
- Python - Generators
- Python - Closures
- Python - Decorators
- Python - Recursion
- Python - Reg Expressions
- Python - PIP
- Python - Database Access
- Python - Weak References
- Python - Serialization
- Python - Templating
- Python - Output Formatting
- Python - Performance Measurement
- Python - Data Compression
- Python - CGI Programming
- Python - XML Processing
- Python - GUI Programming
- Python - Command-Line Arguments
- Python - Docstrings
- Python - JSON
- Python - Sending Email
- Python - Further Extensions
- Python - Tools/Utilities
- Python - GUIs
- Python Advanced Concepts
- Python - Abstract Base Classes
- Python - Custom Exceptions
- Python - Higher Order Functions
- Python - Object Internals
- Python - Memory Management
- Python - Metaclasses
- Python - Metaprogramming with Metaclasses
- Python - Mocking and Stubbing
- Python - Monkey Patching
- Python - Signal Handling
- Python - Type Hints
- Python - Automation Tutorial
- Python - Humanize Package
- Python - Context Managers
- Python - Coroutines
- Python - Descriptors
- Python - Diagnosing and Fixing Memory Leaks
- Python - Immutable Data Structures
- Python Useful Resources
- Python - Questions & Answers
- Python - Interview Questions & Answers
- Python - Online Quiz
- Python - Quick Guide
- Python - Reference
- Python - Cheatsheet
- Python - Projects
- Python - Useful Resources
- Python - Discussion
- Python Compiler
- NumPy Compiler
- Matplotlib Compiler
- SciPy Compiler
Python else Keyword
In Python, the else keyword is used in conditional statements. The else block will be executed only when the if condition is False. This keyword is syntactically dependent on the if keyword. When we use use else keyword without an if statement we will get an SyntaxError.
Syntax
Following is the syntax of Python else keyword −
if condition: statement1 statement2 else: statement3 statement4
Example
Following is a basic example of Python else keyword −
if False: print("Hello") else: print("Hello world")
Output
Following is the output of the above code −
Hello world
Using else Keyword In Functions
The else keyword can also be used in the functions to check conditional statements.
Example
Here, we have defined a function named fun1 to check whether the number is positive or not −
def fun1(num): if num<0: return False else: return True x=9 print(x,"is a positive number :",fun1(x)) y=-4 print(y,"is a positive number :",fun1(y))
Output
Following is the output of the above code −
9 is a positive number : True -4 is a positive number : False
Using else Keyword in Loops
The else keyword is used in loops based on the conditional statements.
Example
Lets try to understand else keyword in loops −
x=[1,2,3,4] for i in x: if i%2==0: print(i,"is a even number in the list") else: print(i,"is not a even number in the list")
Output
Following is the output of the above code −
1 is not a even number in the list 2 is a even number in the list 3 is not a even number in the list 4 is a even number in the list
Using else keyword without if statement
The else keyword depends on if condition. If we will use else block without if condition we will get an SyntaxError.
Example
else: print("Hello")
Output
Following is the output of the above code −
File "E:\pgms\Keywords\else.py", line 28 else: ^^^^ SyntaxError: invalid syntax
Using else keyword with elif
When there are more than one conditional statement to check we can use elif. If all the given conditions are False than else block will be executed.
Example
Here, is an example for usage of else along with elif keyword −
if False: print("Welcome") elif False: print("To") elif False: print("the") else: print("Welcome to Tutorials Point")
Output
Following is the output of the above code −
Welcome to Tutorials Point
Using else with try & except block
We can also use else keyword with try and except block. In this the else block is executed only if try block will not raise any error.
Example
Lets try to execute else along with try and except block −
x = 5 try: x < 10 print("This statement is executed") except: print("Something went wrong") else: print("This statement is executed only if try block is executed without raising any errors")
Output
Following is the output of the above code −
This statement is executed This statement is executed only if try block is executed without raising any errors
Nested else
When there are multiple else within a single else block is known as nested else.
Example
Here, is an example for nested else −
if False: print("This is not executed") else: if False: print("Hello World") else: print("This statement is executed")
Output
Following is the output of the above code −
This statement is executed