Note: If you're new to TypeScript, check our Getting Started with TypeScript tutorial first.
Arrays are collections of elements of the same data type.
Here's a simple example of arrays. You can read the rest of the tutorial to learn more.
Example
let age: number[] = [10, 12, 3, 40, 5];
console.log(age);
Here, age
is an array of numbers representing different ages.
Create an Array
In TypeScript, you create an array by defining a variable with an array type annotation (which specifies the type of elements the array will hold).
For instance, to store the names of students in a class, you'd write:
let studentNames: string[] = ["Alice", "Bob", "Charlie"];
Thus, the syntax to create an array in TypeScript is:
let arrayName: elementType[] = [element1, element2, element3];
Accessing Array Elements
Once an array is created, you can access its elements by their indexes.
The index of an array starts from 0, meaning the first element is at index 0, the second at index 1 and so on. For example,
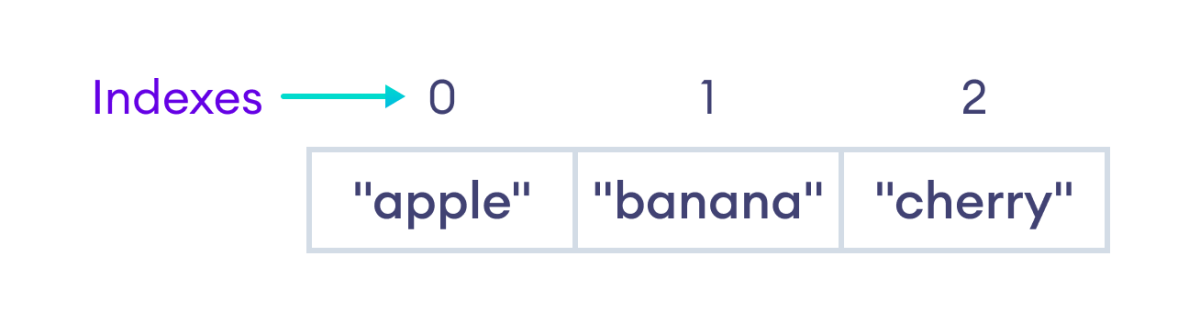
let fruits: string[] = ["apple", "banana", "cherry"];
// Accesses "apple"
let firstFruit: string = fruits[0];
// Accesses "banana"
let secondFruit: string = fruits[1];
console.log(firstFruit);
console.log(secondFruit);
Output
apple banana
Operations in an Array
You can perform various operations on an array, such as adding, removing, and changing its elements.
Let's see each of the operations in detail.
Adding Elements to an Array
You can use the push()
method to add one or more elements to the end of an array. For example:
let fruits: string[] = ["apple", "banana", "cherry"];
// Add "orange" to the end of the array
fruits.push("orange");
console.log(fruits);
Output
["apple", "banana", "cherry", "orange"]
Frequently Asked Questions
You can add one or more elements to the beginning of an array using the unshift()
method. For example,
let fruits: string[] = ["apple", "banana", "cherry"];
fruits.unshift("strawberry");
console.log(fruits);
// Output: ["strawberry", "apple", "banana", "cherry"]
Removing Elements From an Array
You can use the pop()
method to remove the last element from an array. For example,
let fruits: string[] = ["apple", "banana", "cherry"];
let lastFruit: string = fruits.pop();
console.log(lastFruit);
Output
cherry
As you can see, the pop()
method removes the last element and returns it. We then stored this returned value in the lastFruit
variable.
Frequently Asked Questions
You can remove the first element of an array using the shift()
method. For example,
let fruits: string[] = ["apple", "banana", "cherry"];
let firstFruit: string = fruits.shift();
console.log(firstFruit);
// Output: apple
Changing Elements in an Array
You can change an element in an array by assigning a new value to the specified index. For example,
let fruits: string[] = ["apple", "banana", "cherry"];
console.log("Before changing, the second element is" , fruits[1]);
fruits[1] = "mango";
console.log("After changing, the second element is" , fruits[1]);
Output
Before changing, the second element is banana After changing, the second element is mango
Array Methods
There are several other array methods you can use to perform various operations. These methods are summarized in the table below:
Method | Description |
---|---|
concat() |
Joins two or more arrays and returns the result. |
toString() |
Converts an array to a string of comma-separated array values. |
indexOf() |
Searches an element of an array and returns its first index. |
find() |
Returns the value of the first element in the array that satisfies the provided testing function. |
findIndex() |
Returns the first index of the array element that passes a given test. |
forEach() |
Executes a provided function once for each array element. |
includes() |
Checks if an array contains a specified element. |
sort() |
Sorts the elements of an array in place and returns the sorted array. |
slice() |
Selects part of an array and returns it as a new array. |
splice() |
Changes the contents of an array by removing or replacing existing elements and/or adding new elements. |
Example: Array Methods
Let's look at an example to see how some of the above methods work.
let array1: number[] = [1, 2, 3];
let array2: number[] = [4, 5, 6];
// Use concat() to merge array1 and array2
let combinedArray: number[] = array1.concat(array2);
console.log("Combined Array:", combinedArray);
// Use indexOf() to find the index of element '5' in the combinedArray
let index: number = combinedArray.indexOf(5);
console.log('Index of 5:', index);
// Use slice() to get a portion of the combinedArray (elements at index 1 to 3)
let slicedArray: number[] = combinedArray.slice(1, 4);
console.log("Sliced Array:", slicedArray);
// Use splice() to remove two elements starting from index 3 and add '7', '8'
combinedArray.splice(3, 2, 7, 8);
console.log("Modified Combined Array:", combinedArray);
Output
Combined Array: [1, 2, 3, 4, 5, 6] Index of 5: 4 Sliced Array: [2, 3, 4] Modified Combined Array: [1, 2, 3, 7, 8, 6]
Frequently Asked Questions
You can also create an array using the new
keyword. For example,
1. Create an empty array.
let emptyArray: number[] = new Array();
2. Create an array of specified length.
// Creates an array with 5 empty slots.
let arrayWithSize: number[] = new Array(5);
3. Create an array with elements.
let arrayWithElements: number[] = new Array(1, 2, 3, 4);
Also Read: