Note: If you're new to TypeScript, check our Getting Started with TypeScript tutorial first.
TypeScript strings are a primitive data type used to represent textual data.
Here's a simple example of TypeScript strings. You can read the rest of the tutorial for more.
Example
let message: string = "Good Morning!";
console.log(message);
// Output: Good Morning!
Here, message
is a variable of string
type and is initialized with the value "Good Morning!"
.
Declare TypeScript Strings
The syntax to declare a string in TypeScript is:
let variableName: string;
For example,
let greetings: string;
You can assign a value to greetings
using single quotes, double quotes, or backticks:
- Single Quotes:
greetings = 'Hello';
- Double Quotes:
greetings = "Hello";
- Backticks:
greetings = `Hello`;
Single Quotes and Double Quotes Are Identical
Single quotes and double quotes function identically in TypeScript and can be used interchangeably.
// String enclosed within single quotes
let language: string = 'English';
console.log(language); // English
// String enclosed within double quotes
let country: string = "USA";
console.log(country); // USA
Frequently Asked Questions
When writing strings in TypeScript, you must ensure that if you start a string with a single quote ('
), you must also end it with a single quote and vice versa.
The following code will throw a syntax error:
let greeting: string = 'Hello, world!";
Backticks Allow Variable and Expressions
Unlike single and double quotes, backticks allow the insertion of variables or expressions directly into the string.
// String enclosed within backticks
let userName: string = "Jack";
console.log(`Hello, ${userName}`);
In this example, we've used backticks to create a string that directly includes the variable userName
.
Output
Hello, Jack
Access String Characters
You can access individual characters of a string using the bracket notation []
. Here's how you can do it:
let greeting: string = "Hello";
// Access the first character, 'H'
let firstCharacter = greeting[0];
console.log(firstCharacter);
// Access the second character, 'e'
let secondCharacter = greeting[1];
console.log(secondCharacter);
Output
H e
Each character in a string can be accessed by its index, starting from 0 for the first character, 1 for the second character and so on.
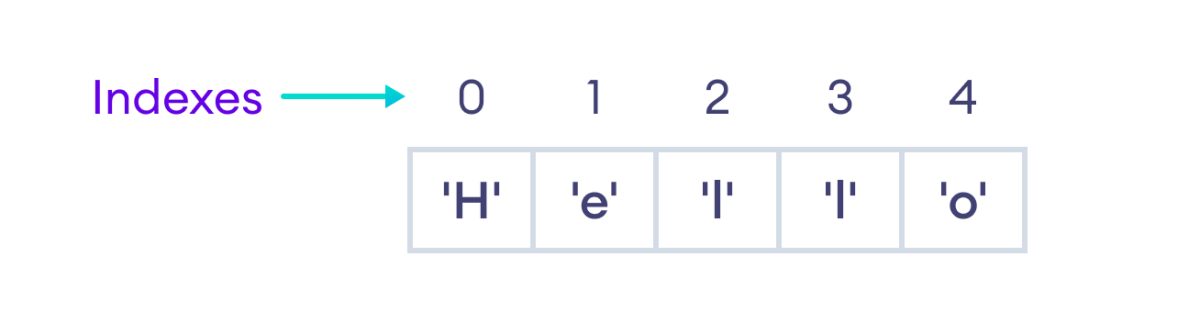
Features of String
Just like JavaScript strings, TypeScript strings have several notable features that are important for programming.
1. Strings are Immutable
TypeScript strings are immutable. This means that once a string is created, the characters within it cannot be changed. For example,
let message: string = "hello";
message[0] = "H";
console.log(message);
// Output: Index signature in type 'String' only permits reading.
In the example above, we attempted to change the first character of message
using message[0] = "H";
. However, this operation does not alter the string due to its immutable nature.
While you cannot modify the existing characters of a string, you can always assign a new value to the string variable itself. For example,
let message: string = "hello";
message = "Hello";
console.log(message); // Outputs: Hello
2. Case Sensitivity
TypeScript strings are case-sensitive, meaning that lowercase and uppercase letters are considered distinct. For example,
let value1: string = "a";
let value2: string = "A";
// Check if value1 and value2 are strictly equal
console.log(value1 === value2); // Output: false
Here, value1
and value2
are treated as different because 'a'
and 'A'
are not the same regarding casing.
String Methods
There are string methods you can use to perform operations on strings. They are:
Method | Description |
---|---|
charAt() |
Returns the character at the specified index. |
concat() |
Joins two or more strings. |
replace() |
Replace a string with another string. |
split() |
Converts the string to an array of strings. |
substr() |
Returns a part of a string by taking the starting position and length of the substring. |
substring() |
Returns a part of the string from the specified start index (inclusive) to the end index (exclusive). |
slice() |
Returns a part of the string from the specified start index (inclusive) to the end index (exclusive). |
toLowerCase() |
Returns the passed string in lowercase. |
toUpperCase() |
Returns the passed string in uppercase. |
trim() |
Removes whitespace from the strings. |
includes() |
Searches for a string and returns a boolean value. |
search() |
Searches for a string and returns the position of a match. |
Example: String Methods
// Initial string declaration
let baseString: string = " Hello World!";
// Using trim() to ensure there are no leading or trailing whitespaces
let trimmedString: string = baseString.trim();
// Using toUpperCase() to convert the entire string to uppercase
let upperCaseString: string = trimmedString.toUpperCase();
// Using replace() to replace "WORLD" with "TS"
let replacedString: string = upperCaseString.replace("WORLD", "TS");
// Using slice() to extract a part of the string
let sliceOfString: string = replacedString.slice(0, 5); // Extracts 'HELLO'
// Using charAt() to find the first character of the trimmed string
let firstChar: string = trimmedString.charAt(0); // 'H'
console.log(`Original string: ${baseString}`);
console.log(`Trimmed: ${trimmedString}`);
console.log(`Uppercase: ${upperCaseString}`);
console.log(`Replaced "WORLD" with "TS": ${replacedString}`);
console.log(`Slice of the first part: ${sliceOfString}`);
console.log(`First character: ${firstChar}`);
Output
Original string: Hello World! Trimmed: Hello World! Uppercase: HELLO WORLD! Replaced "WORLD" with "TS": HELLO TS! Slice of the first part: HELLO First character: H
Note: Since strings are immutable in TypeScript, these methods don't modify the original string; instead, they return a new one.
Frequently Asked Questions
You can retrieve the length of a string using the length
property on the string variable. For example,
let message: string = "Hello, TypeScript!";
let messageLength: number = message.length;
console.log(messageLength);
// Output: 18
In TypeScript, strings can be treated both as primitive values and as objects.
String primitives: String primitives are the most commonly used form of strings. For example,
let greeting: string = "Hello, world!";
String Object: TypeScript also allows strings to be defined as objects using the String()
constructor. For example,
let objectString: String = new String("Hello, world!");
Also Read: