Note: If you're new to TypeScript, check our Getting Started with TypeScript tutorial first.
A constructor is a special method of a class that is automatically called when you create a new instance of that class using the new keyword.
It is mainly used to initialize the properties of the new object.
Here's a simple example of a class constructor. You can read the rest of the tutorial to learn more.
Example
class Student {
name: string;
constructor(name: string) {
this.name = name; // Initialize the property
console.log("Constructor is called");
}
greet(): string {
return `Welcome, ${this.name}!`;
}
}
// Create an instance of Student class
// Pass "Leon Kennedy" as argument to the constructor
let student1 = new Student("Leon Kennedy");
console.log(student1.greet());
// Output:
// Constructor is called
// Welcome, Leon Kennedy!
Here, constructor()
is automatically called the moment we create the student1
object. It initializes the name
property to "Leon Kennedy"
.
Creating a Constructor
We use the constructor
keyword to create a constructor inside a class. Here's the syntax on how to do it:
class ClassName {
constructor(parameters) {
// Initialization code
}
}
Here,
ClassName
- The name of the class whose constructor you're creating.constructor
- Keyword to create the constructor. This keyword also acts as the name of the constructor method.parameters
- Constructor parameters whose values are used for initializing the class properties.
Notes:
- The constructor method must always be named as
constructor
. - A class can have only one constructor.
Example 1: TypeScript Constructor Method
class Student {
name: string;
gpa: number;
constructor(name: string, gpa: number) {
// Initialize the properties
this.name = name;
this.gpa = gpa;
}
}
// Create an instance of Student class
// Pass "Leon Kennedy" and 3.8 as arguments to its constructor
let student1 = new Student("Leon Kennedy", 3.8);
// Print the name and gpa properties of student1
console.log(`Name: ${student1.name}`);
console.log(`GPA: ${student1.gpa}`);
Output
Name: Leon Kennedy GPA: 3.8
Here, the Student
class has two properties: name
and gpa
.
Our constructor method initializes these properties by assigning the values of its arguments to the respective class properties:
constructor(name: string, gpa: number) {
// Initialize the properties
this.name = name;
this.gpa = gpa;
}
Inside the constructor method,
name
andgpa
- The parameters of the constructor.this.name
andthis.gpa
- The instance properties we declared in the class (above the constructor). More specifically,this.name
andthis.gpa
refer to the properties belonging to each individual instance of the class.
Having defined our class, we then created an instance of the Student
class (student1
) by passing the required arguments to its constructor:
let student1 = new Student("Leon Kennedy", 3.8);
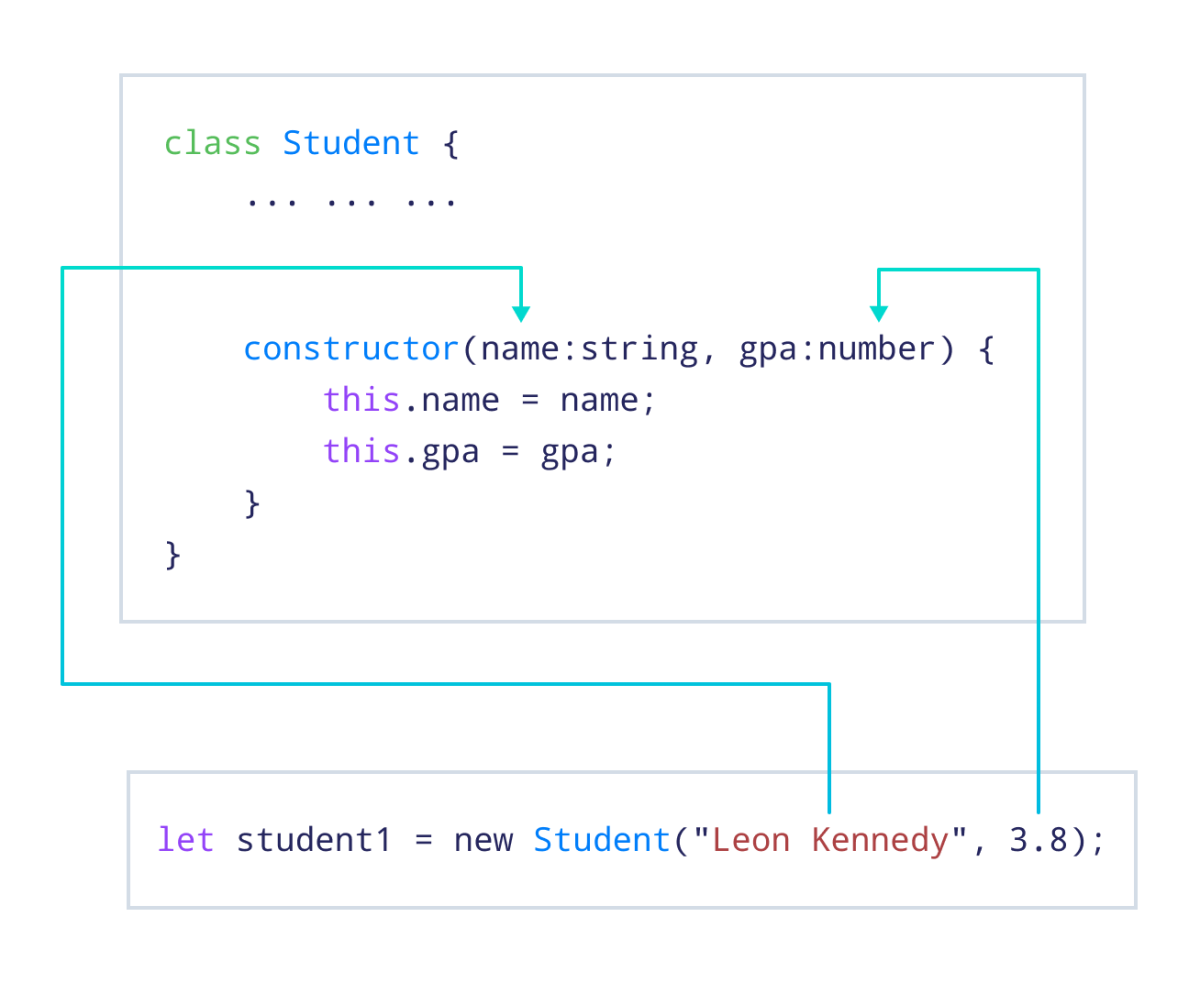
Example 2: Creating Multiple Instances
Now, let's rewrite the previous program to create two instances of the Student
class.
We'll also create a printInfo()
method to print the name
and gpa
properties of the objects.
class Student {
name: string;
gpa: number;
constructor(name: string, gpa: number) {
// Initialize the properties
this.name = name;
this.gpa = gpa;
}
// Method to print student details
printInfo(): void {
console.log(`Name: ${this.name}`);
console.log(`GPA: ${this.gpa}`);
console.log();
}
}
// Create an instance of Student class
// Pass "Leon Kennedy" and 3.8 as arguments to its constructor
let student1 = new Student("Leon Kennedy", 3.8);
// Create another instance of Student class
// Pass "Ada Wong" and 3.6 as arguments to its constructor
let student2 = new Student("Ada Wong", 3.6);
// Call the printInfo() method of the two instances
student1.printInfo();
student2.printInfo();
Output
Name: Leon Kennedy GPA: 3.8 Name: Ada Wong GPA: 3.6
Here, we've created two instances of the Student
class (student1
and student2
) by passing the required arguments to their constructors:
let student1 = new Student("Leon Kennedy", 3.8);
let student2 = new Student("Ada Wong", 3.6);
1. When student1 is created.
- The
name
parameter has the value"Leon Kennedy"
. - The
gpa
parameter has the value 3.8.
2. When student2 is created.
- The
name
parameter has the value"Ada Wong"
. - The
gpa
parameter has the value 3.6.
Constructor Parameter Properties (Shorthand)
You can also declare and initialize properties directly in the constructor parameters by adding visibility modifiers such as public
, private
, protected
, or readonly
. For example,
class Student {
constructor(public name: string, private gpa: number) {}
getGPA(): number {
return this.gpa;
}
}
let student1 = new Student("Leon Kennedy", 3.8);
console.log(`Name: ${student1.name}`);
console.log(`GPA: ${student1.getGPA()}`);
Output
Name: Leon Kennedy GPA: 3.8
As you can see, we've declared and initialized two properties in the constructor:
public name: string
- Declares and initializes apublic
propertyname
.private gpa: number
- Declares and initializes aprivate
propertygpa
.
Note: Using this shorthand reduces boilerplate code.
The above program is equivalent to the program below:
class Student {
public name: string;
private gpa: number;
constructor(name: string, gpa: number) {
this.name = name;
this.gpa = gpa;
}
getGPA(): number {
return this.gpa;
}
}
let student1 = new Student("Leon Kennedy", 3.8);
console.log(`Name: ${student1.name}`);
console.log(`GPA: ${student1.getGPA()}`);
More on TypeScript Constructors
You can also provide default values for constructor parameters:
class Student {
constructor(public name: string = "Unknown", public gpa: number = 0) {}
}
// Don't pass any value to the constructor
let student1 = new Student();
// Pass values to the constructor
let student2 = new Student("Jill Valentine", 4);
console.log(`Student1 Name: ${student1.name}`);
console.log(`Student1 GPA: ${student1.gpa}`);
console.log(`Student2 Name: ${student2.name}`);
console.log(`Student2 GPA: ${student2.gpa}`);
Output
Student1 Name: Unknown Student1 GPA: 0 Student2 Name: Jill Valentine Student2 GPA: 4
If no arguments are passed, the default values are used.
When you derive one class from another, the derived class must call super()
in its constructor to invoke the constructor of the base class.
class Person {
constructor(public name: string) {}
}
class Student extends Person {
constructor(name: string, public gpa: number) {
// Call constructor of Person class
super(name);
}
getInfo(): string {
return `${this.name} has a GPA of ${this.gpa}`;
}
}
let student1 = new Student("Leon Kennedy", 3.8);
console.log(student1.getInfo());
// Output: Leon Kennedy has a GPA of 3.8
Here, the Student
class is derived from the Person
class. Thus, Student
objects can access Person
's properties and constructor.
In the Student
class, we've used super()
inside its constructor()
method to access the constructor of the Person
class. This ensures that the name
property is also initialized.
Read More: