Note: If you're new to TypeScript, check our Getting Started with TypeScript tutorial first.
TypeScript allows you to pass default values to a function. They are used when the function is called without passing the corresponding arguments.
Example
function greet(name: string = "Guest"): void {
console.log(`Hello, ${name}!`);
}
greet();
// Output: Hello, Guest!
In this example, the greet()
function has a default parameter name
with the string value "Guest"
. Since we have not passed any argument to the function, it uses the default value.
Example 1: TypeScript Default Parameters
function sum(x: number = 3, y: number = 5): number {
// Return sum
return x + y;
}
// Pass arguments to x and y
let result: number = sum(5, 15);
console.log(`Sum of 5 and 15: ${result}`);
// Pass argument to x but not to y
result = sum(7);
console.log(`Sum of 7 and default value (5): ${result}`);
// Pass no arguments
// Use default values for x and y
result = sum();
console.log(`Sum of default values (3 and 5): ${result}`);
Output
Sum of 5 and 15: 20 Sum of 7 and default value (5): 12 Sum of default values (3 and 5): 8
In the above example, the default value of x is 3 and the default value of y is 5.
sum(5, 15)
- When both arguments are passed, x takes 5 and y takes 15.sum(7)
- When 7 is passed, x takes 7 and y takes the default value 5.sum()
- When no argument is passed, x and y take the default values 3 and 5, respectively.
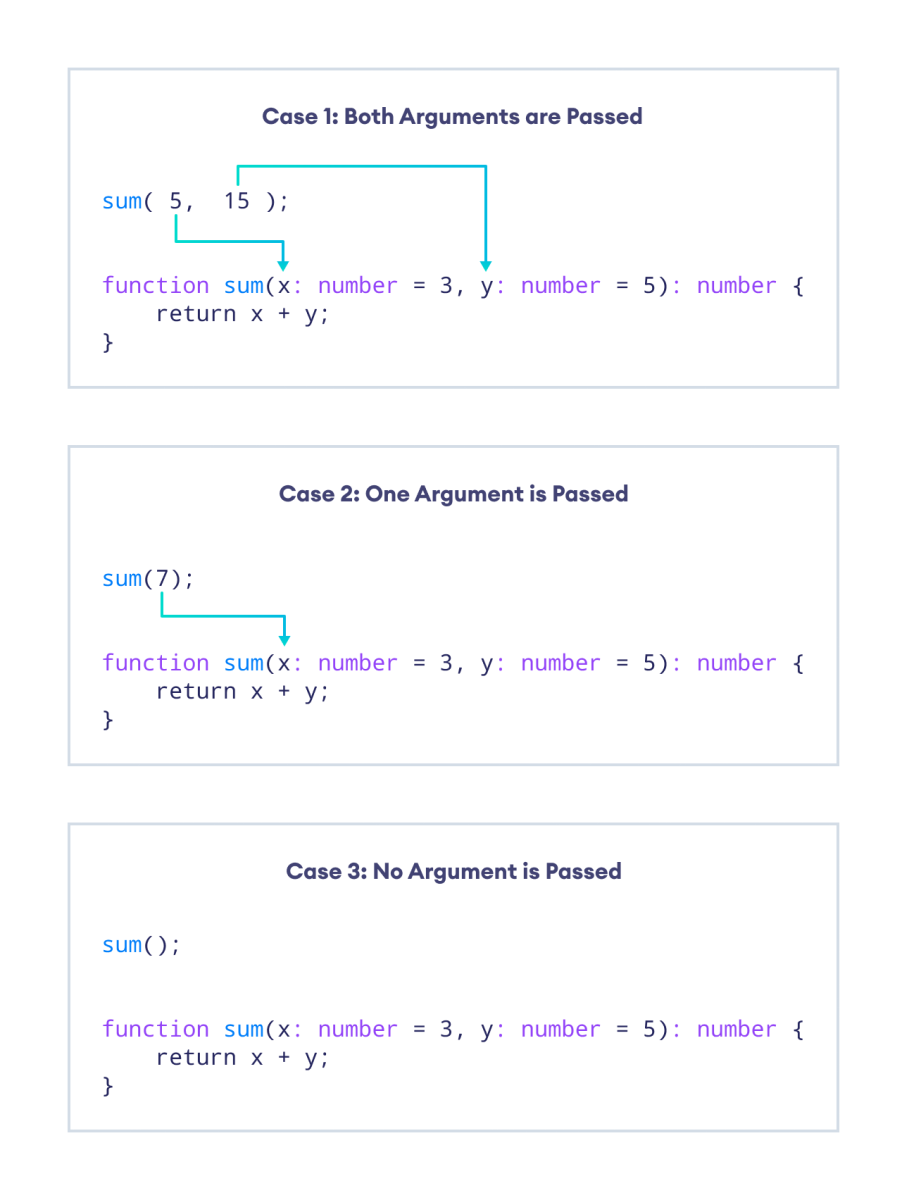
Default Parameters Should Come After Required Parameters
If your function has both default and required parameters (i.e., those without default values), you should place the default parameters after the required ones. For example,
function sum(x: number, y: number, z: number = 10): number {
return x + y + z;
}
// Don't pass argument to z
// So z = 10 by default
let result: number = sum(5, 15);
console.log(`5 + 15 + 10 = ${result}`);
// Pass argument to z
result = sum(5, 15, 13);
console.log(`5 + 15 + 13 = ${result}`);
Output
5 + 15 + 10 = 30 5 + 15 + 13 = 33
In the above example, we've kept the default parameter z: number = 10
at the end of the parameter list.
As a result, when TypeScript encounters the code sum(5, 15)
, it clearly knows that x = 5
and y = 15
.
Keeping Default Arguments at the Beginning
Now, let's write a similar program by keeping the default parameter at the beginning:
function sum(x: number = 10, y: number, z: number): number {
return x + y + z;
}
// Only pass two arguments
// Invalid code
let result: number = sum(5, 15);
console.log(result);
Output
error: Expected 3 arguments, but got 2. An argument for 'z' was not provided.
As you can see, we got an error when we passed only two arguments to the sum()
function.
It's because TypeScript assigns 5 to x
and 15 to y
. Thus, no argument is passed to the z
parameter, which causes an error.
Note: You'll face similar problems if you place your default parameters between required parameters.
More on Default Parameters
In TypeScript, you can pass one parameter as the default value for another. For example,
function sum(x: number = 1, y: number = x, z: number = x + y): void {
console.log(x + y + z);
}
sum();
// Output: 4
In the above example,
- The default value of x is 1.
- The default value of y is set to the x parameter.
- The default value of z is the sum of x and y.
So when sum()
is called without any arguments, it uses these default values, leading to the calculation 1 + 1 + 2 = 4
. Hence, the output is 4.
We can also pass a function as a default value in TypeScript. For example,
function sum(): number {
return 15;
}
// Use sum() in default value of y
function calculate( x, y = x * sum() ): number {
return x + y;
}
let result: number = calculate(10);
console.log(result);
// Output: 160
Here,
- The
sum()
function returns 15. - 10 is passed to the
calculate()
function. - x becomes 10, and y becomes 150 (
x * sum()
). - The result will be 160 (
x + y
).
In TypeScript, when you pass undefined
to a default parameter, the function takes the default value. For example,
function test(x: number = 1): void {
console.log(x);
}
// Pass undefined
// Takes default value 1
test(undefined);
// Output: 1
This is also applicable for functions that have default parameters in the beginning or in the middle of the parameter list. For example,
// Function that has default parameter at the beginning
function sum(x: number = 10, y: number, z: number): number {
return x + y + z;
}
// Function that has default parameter at the middle
function difference(x: number, y: number = 10, z: number): number {
return x - y - z;
}
// Call sum() function
// Pass undefined to default parameter
let result: number = sum(undefined, 5, 15);
console.log(`10 + 5 + 15 = ${result}`);
// Call difference() function
// Pass undefined to default parameter
result = difference(5, undefined, 15);
console.log(`5 - 10 - 15 = ${result}`);
// Output:
// 10 + 5 + 15 = 30
// 5 - 10 - 15 = -20
Also Read: