Note: If you're new to TypeScript, check our Getting Started with TypeScript tutorial first.
TypeScript classes provide a blueprint for creating objects with predefined properties and methods.
Here's a simple example of classes. You can read the rest of the tutorial to learn more.
Example
// Define a class named 'Person'
class Person {
name: string;
age: number;
// Constructor to initialize 'name' and 'age'
constructor(name: string, age: number) {
this.name = name;
this.age = age;
}
// Method to display a message
greet(): void {
console.log(`My name is ${this.name} and I am ${this.age} years old.`);
}
}
// Create instances of the Person class
let person1 = new Person("Jack", 30);
let person2 = new Person("Tina", 33);
// Call greet() method
person1.greet();
person2.greet();
// Output
// My name is Jack and I am 30 years old.
// My name is Tina and I am 33 years old.
Here, we created a Person
class with a constructor to initialize name
and age
, and a greet()
method to print a message. Then, we created two objects and called the method on each.
Create Objects Without Classes
In TypeScript, you have the flexibility to create objects directly without the use of formal class definitions. This can be achieved by using object literals.
Let's look at the example below,
// Create an object 'person' with type annotation
let person: {
name: string;
age: number;
greet: () => void;
} = {
name: "Jack",
age: 30,
greet() {
console.log(`My name is ${this.name} and I am ${this.age} years old.`);
}
};
// Call the greet() method on the person object
person.greet();
Output
My name is Jack and I am 30 years old.
In the above example, we have created an object named person
directly using an object literal.
The person
object has:
- properties -
name
andage
with values"Jack"
and 30 respectively. - method -
greet()
that displays a greeting message.
We have called the greet()
method on person
using the .
operator as person.greet()
.
To learn more about object literals, visit TypeScript Objects.
Features of a TypeScript Class
Let's revisit the code from the beginning of the tutorial and explore each part in detail to gain a deeper understanding of how classes work in TypeScript.
// Define a class named 'Person'
class Person {
name: string;
age: number;
// Constructor to initialize 'name' and 'age'
constructor(name: string, age: number) {
this.name = name;
this.age = age;
}
// Method to display a message
greet(): void {
console.log(`My name is ${this.name} and I am ${this.age} years old.`);
}
}
// Create instances of the Person class
let person1 = new Person("Jack", 30);
let person2 = new Person("Tina", 33);
// Call greet() method
person1.greet();
person2.greet();
Output
My name is Jack and I am 30 years old. My name is Tina and I am 33 years old
Create a class
In TypeScript, we create a class using the class
keyword. For example,
// Create a class
class Person {
// Body of class
};
Class Constructor
A constructor is a special method in a class that runs automatically when an instance is created using the new
keyword.
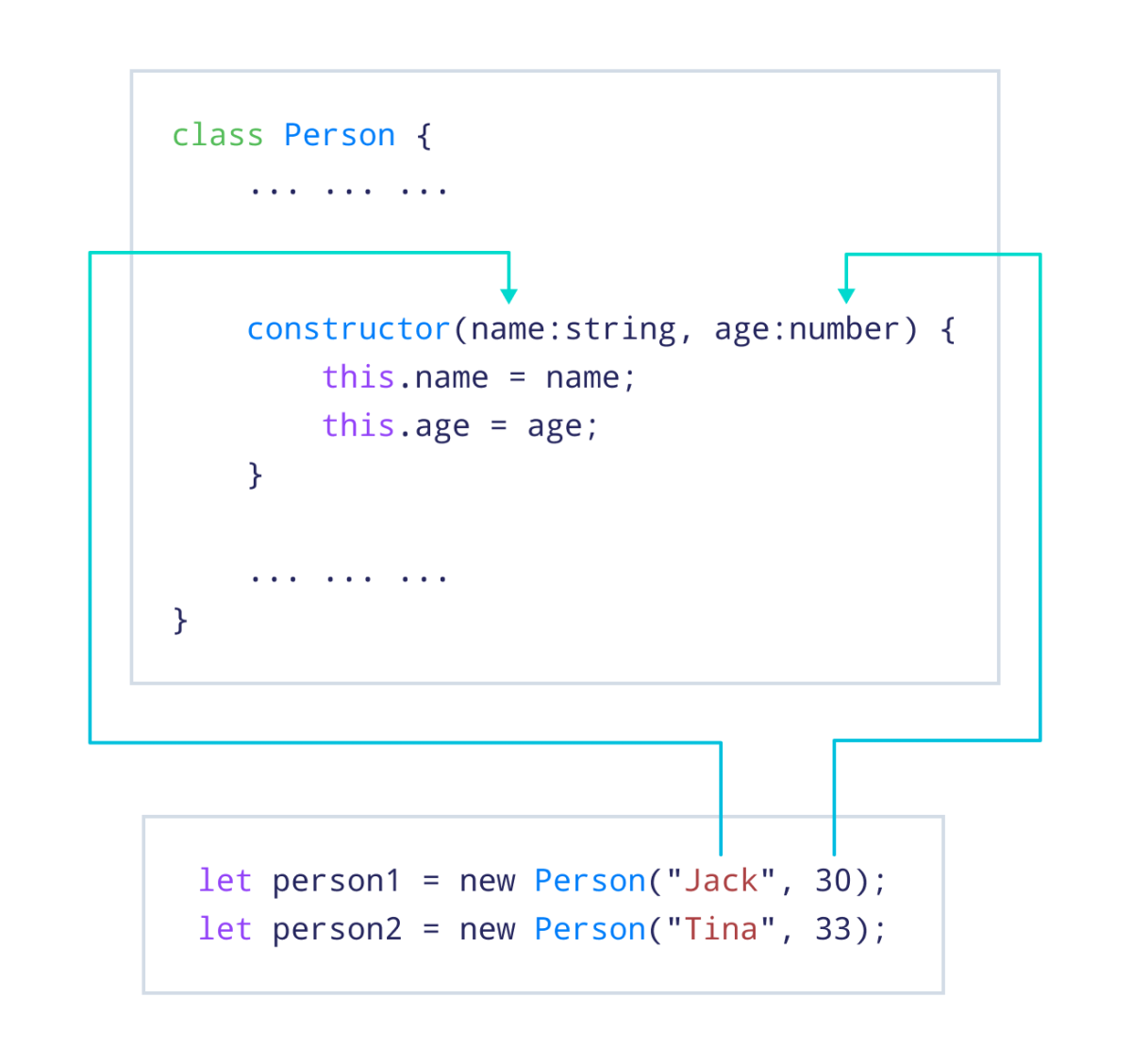
The Person
class constructor initializes the name
and age
properties when a new instance of the class is created.
Here,
- the
person1
object is initialized with"Jack"
and 30 - the
person2
object is initialized with"Tina"
and 33
Class Method
A class method is a function inside a class that defines behaviors for the class's objects.
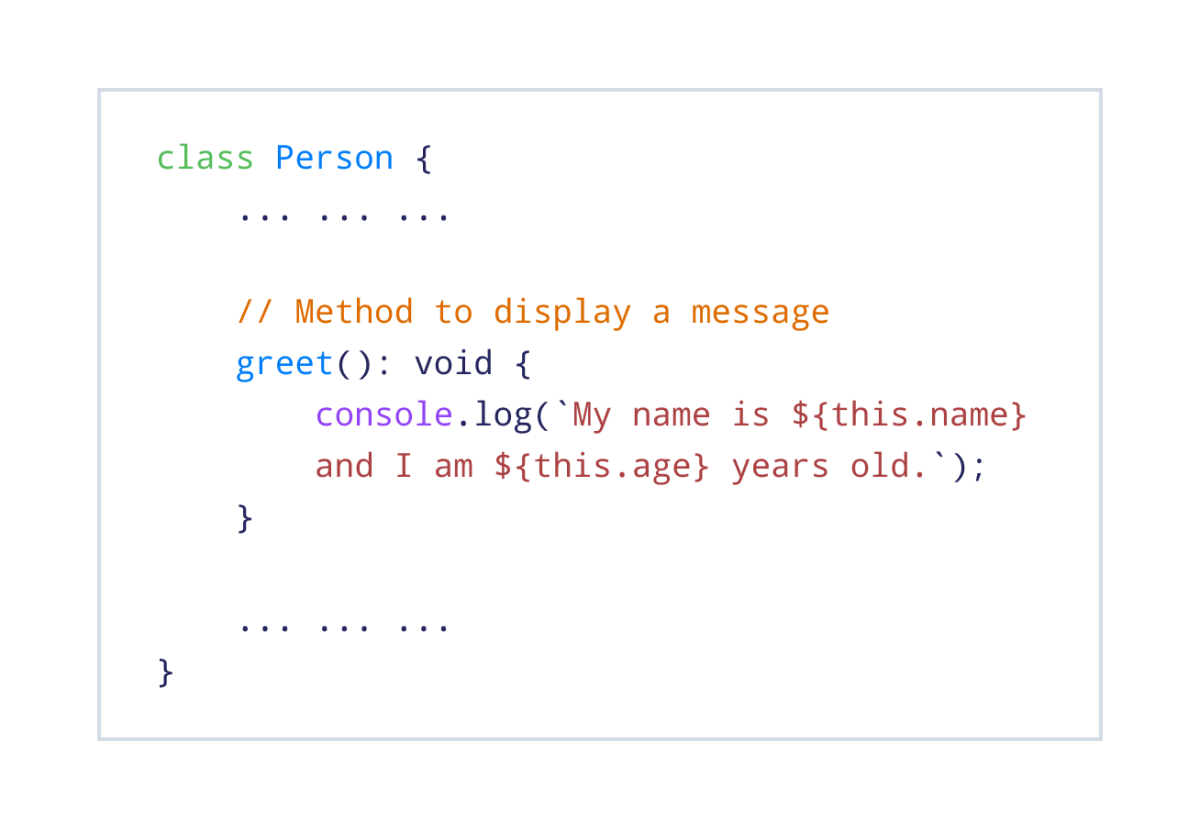
Here, greet()
is a method of the Person
class that displays a greeting message when called on objects of the class.
More on TypeScript Classes
In TypeScript, you can directly assign default values to class properties. That way, even if no values are passed when creating an object, the defaults will be used. For example,
class Person {
name: string = "Jack";
age: number = 18;
greet(): void {
console.log(`My name is ${this.name} and I am ${this.age} years old.`);
}
}
let person1 = new Person();
person1.greet();
// Output: My name is Jack and I am 18 years old.
Here, name
and age
already have values. So even though we didn't pass anything when creating person1
, it still works using the default values.
When assigning default values, TypeScript can automatically infer the type of a property. For example,
class Animal {
type = "Dog"; // TypeScript infers: type: string
}
let pet = new Animal();
console.log(typeof pet.type); // Output: string
In TypeScript, access modifiers control where class properties or methods can be accessed:
public
- can be accessed anywhere (default)private
- can only be used inside the classprotected
- can be used inside the class and its subclasses
For example,
class Person {
public name: string = "Jack";
private age: number = 30;
}
let p1 = new Person();
console.log(p1.name); // Output: Jack
console.log(p1.age); // Error: Property 'age' is private
Here, name
is public and accessible directly. But age
is private — so using it outside the class causes an error.
To learn more, refer to TypeScript Access Modifiers.
Read More: