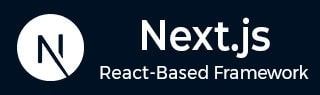
- Next.js - Home
- Next.js - Overview
- Next.js - Project Setup
- Next.js - Folder Structure
- Next.js - App Router
- Next.js - Page Router
- Next.js Features
- Next.js - Pages
- Next.js - Data Fetching
- Next.js - ISR
- Next.js - Static File Serving
- Next.js - Pre-Rendering
- Next.js - Partial Pre Rendering
- Next.js - Server Side Rendering
- Next.js - Client Side Rendering
- Next.js Routing
- Next.js - Routing
- Next.js - Nested Routing
- Next.js - Dynamic Routing
- Next.js - Parallel Routing
- Next.js - Imperative Routing
- Next.js - Shallow Routing
- Next.js - Intercepting Routes
- Next.js - Redirecting Routes
- Next.js - Navigation and Linking
- Next.js Configuration
- Next.js - TypeScript
- Next.js - Environment Variables
- Next.js - File Conventions
- Next.js - ESLint
- Next.js API & Backend
- Next.js - API Routes
- Next.js - Dynamic API Routes
- Next.js - Route Handlers
- Next.js - API MiddleWares
- Next.js - Response Helpers
- Next.js API Reference
- Next.js - CLI Commands
- Next.js - Functions
- Next.js - Directives
- Next.js - Components
- Next.js - Image Component
- Next.js - Font Component
- Next.js - Head Component
- Next.js - Form Component
- Next.js - Link Component
- Next.js - Script Component
- Next.js Styling & SEO
- Next.js - CSS Support
- Next.js - Global CSS Support
- Next.js - Meta Data
- Next.js Advanced Topics
- Next.js - Error Handling
- Next.js - Server Actions
- Next.js - Fast Refresh
- Next.js - Internationalization
- Next.js - Authentication
- Next.js - Session Management
- Next.js - Authorization
- Next.js - Caching
- Next.js - Data Caching
- Next.js - Router Caching
- Next.js - Full Route Caching
- Next.js - Request Memoization
- Next.js Performance Optimization
- Next.js - Optimizations
- Next.js - Image Optimization
- Next.js - Lazy Loading
- Next.js - Font Optimization
- Next.js - Video Optimization
- Next.js - Script Optimization
- Next.js - Memory Optimization
- Next.js - Using OpenTelemetry
- Next.js - Package Bundling Optimization
- Next.js Testing
- Next.js - Testing
- Next.js - Testing with Jest
- Next.js - Testing with Cypress
- Next.js - Testing with Vitest
- Next.js - Testing with Playwright
- Next.js Debugging & Deployment
- Next.js - Debugging
- Next.js - Deployment
- Next.js Useful Resources
- Next.js - Interview Questions
- Next.js - Quick Guide
- Next.js - Useful Resources
- Next.js - Discussion
Next.js - CLI dev Command
In Next.js CLI, the `dev' command is used to setup developmental server for Next.js application. This command have several options to customize behavior or development environment. In the chapter we will explain how to use `dev` command to setup customizable Next.js environment.
Next.js Dev Command Syntax
Following syntax of dev command in Next.js CLI.
npx next dev [options]
For example, `npx next dev -p 3001`, Here port number 3001 is an `option` of `dev` command.
Options of Dev Command
Following are list of options available for `dev` command.
Options | Explanation |
---|---|
-h, --help | List down all available options. |
[directory] | Used to specify directory to build the application. If not provided, current directory is used. |
--turbopack | Starts development mode using Turbopack. |
-p or --port | Specify a port number on which to start the application. Default: 3000, env: PORT |
-H or --hostname | Specify a hostname on which to start the application. Useful for making the application available for other devices on the network. Default: 0.0.0.0 |
--experimental-https | Starts the server with HTTPS and generates a self-signed certificate. |
--experimental-https-key | Specify path to a HTTPS key file. |
--experimental-https-cert | Specify path to a HTTPS certificate file. |
--experimental-https-ca | Specify path to a HTTPS certificate authority file. |
--experimental-upload-trace | Reports a subset of the debugging trace to a remote HTTP URL. |
Change Default Hostname Using Dev Command
In Next.js, we can use the dev command to change the default hostname of the development server. Following is the syntax to change the default hostname.
npx next dev -H 127.0.35.1
After running the above command in your terminal, the Next.js development mode will start running on `http://127.0.35.1:3000/` by default. Your default browser will open the page automatically.
Example
Once your server is ready, paste the below code into the `app/page.tsx` file to see your website running on the updated hostname.
// app/page.tsx export default function Home() { return ( <div> <h1>Welcome to My Next.js App</h1> <p>This is the home page.</p> </div> ); }
Output
In output, you can see that Next.js server is running on host 127.0.35.1.

Change Default Port Using Dev Command
In Next.js we can use dev command to change default port of development server. Following is syntax to change default port number.
npx next dev -p 4000
After running the above command in your terminal, Next.js development mode will start running on `http://localhost:4000/`. Your default browser will open the page automatically.
Example
Once your server is ready, paste the below code on `app/page.tsx` file to see your website running on port 4000.
// app/page.tsx export default function Home() { return ( <div> <h1>Welcome to My Next.js App</h1> <p>This is the home page.</p> </div> ); }
Output
In output, you can see that Next.js server is running on port 4000.
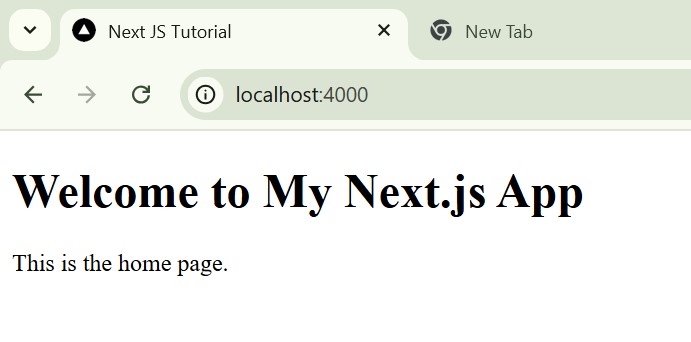