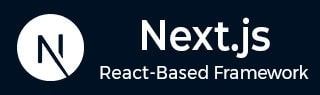
- Next.js - Home
- Next.js - Overview
- Next.js - Project Setup
- Next.js - Folder Structure
- Next.js - App Router
- Next.js - Page Router
- Next.js Features
- Next.js - Pages
- Next.js - Data Fetching
- Next.js - ISR
- Next.js - Static File Serving
- Next.js - Pre-Rendering
- Next.js - Partial Pre Rendering
- Next.js - Server Side Rendering
- Next.js - Client Side Rendering
- Next.js Routing
- Next.js - Routing
- Next.js - Nested Routing
- Next.js - Dynamic Routing
- Next.js - Parallel Routing
- Next.js - Imperative Routing
- Next.js - Shallow Routing
- Next.js - Intercepting Routes
- Next.js - Redirecting Routes
- Next.js - Navigation and Linking
- Next.js Configuration
- Next.js - TypeScript
- Next.js - Environment Variables
- Next.js - File Conventions
- Next.js - ESLint
- Next.js API & Backend
- Next.js - API Routes
- Next.js - Dynamic API Routes
- Next.js - Route Handlers
- Next.js - API MiddleWares
- Next.js - Response Helpers
- Next.js API Reference
- Next.js - CLI Commands
- Next.js - Functions
- Next.js - Directives
- Next.js - Components
- Next.js - Image Component
- Next.js - Font Component
- Next.js - Head Component
- Next.js - Form Component
- Next.js - Link Component
- Next.js - Script Component
- Next.js Styling & SEO
- Next.js - CSS Support
- Next.js - Global CSS Support
- Next.js - Meta Data
- Next.js Advanced Topics
- Next.js - Error Handling
- Next.js - Server Actions
- Next.js - Fast Refresh
- Next.js - Internationalization
- Next.js - Authentication
- Next.js - Session Management
- Next.js - Authorization
- Next.js - Caching
- Next.js - Data Caching
- Next.js - Router Caching
- Next.js - Full Route Caching
- Next.js - Request Memoization
- Next.js Performance Optimization
- Next.js - Optimizations
- Next.js - Image Optimization
- Next.js - Lazy Loading
- Next.js - Font Optimization
- Next.js - Video Optimization
- Next.js - Script Optimization
- Next.js - Memory Optimization
- Next.js - Using OpenTelemetry
- Next.js - Package Bundling Optimization
- Next.js Testing
- Next.js - Testing
- Next.js - Testing with Jest
- Next.js - Testing with Cypress
- Next.js - Testing with Vitest
- Next.js - Testing with Playwright
- Next.js Debugging & Deployment
- Next.js - Debugging
- Next.js - Deployment
- Next.js Useful Resources
- Next.js - Interview Questions
- Next.js - Quick Guide
- Next.js - Useful Resources
- Next.js - Discussion
Next.js - Optimize Memory Usage
Memory Usage in Next.js
Next.js applications use system memory to store and manage data of the application. The memory usage of Next.js application can be divided into two parts, server side memory and client side memory.
Server Side Memory
Next.js application use server side memory for:
- Server-side rendering (SSR)
- API routes execution
- Data fetching and caching
- WebSocket connections
Client Side Memory
Next.js application use client side memory for:
- DOM elements and event listeners
- Browser caching
- JavaScript bundle size
- Media assets and resources
Monitor Next.js Memory Usage
Next.js provides a built-in tools to monitor memory usage of the application. The section below explains how to install and use the memory monitoring tools in Next.js.
Display Memory Usage on Application Build
The Next.js version 14 and above provide a feature to display memory usage on application build. You can run 'next build --experimental-debug-memory-usage' to run the build in a mode where Next.js will print out information about memory usage continuously throughout the build, such as heap usage and garbage collection statistics. The image below shows the output of the memory usage.
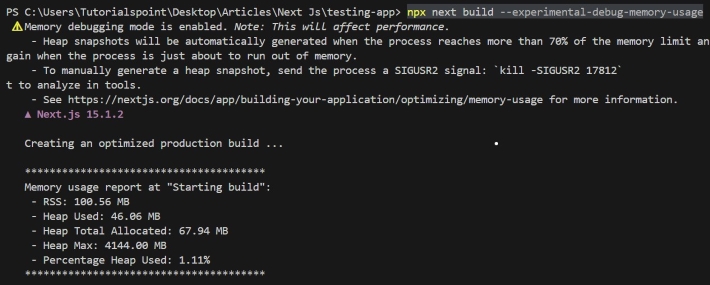
Record a Heap Profile
If you want look further into memory issues, you can record a heap profile from Node.js and load it in Chrome DevTools to identify potential sources of memory leaks. For this you have to pass the --heap-prof flag to Node.js when starting your Next.js build. Use the following command to start build.
node --heap-prof node_modules/next/dist/bin/next build
At the end of the build, a .heapprofile file will be created by Node.js. You can start production server and run your application now. In Chrome DevTools, you can open the Memory tab and click on the "Load Profile" button to visualize the file. See the image below.
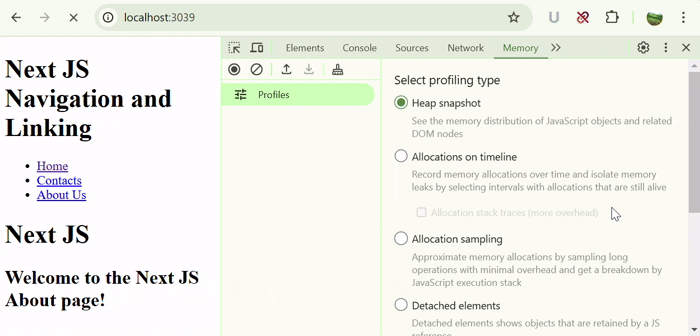
Techniques to Optimize Memory Usage
- Reduce JavaScript Bundles: Next.js application may contain lots of JavaScript files that are not useful for the initial page load. You can activate tree shaking to optimize the bundle size.
- Use Optimized Imports: We can optimize the importing of large packages by adding the 'optimizePackageImports' option to our 'next.config.js' file
- Disable Source Maps: Generating source maps consumes extra memory during the build process. You can disable source map generation by adding 'productionBrowserSourceMaps: false' and 'experimental.serverSourceMaps: false' to your Next.js configuration.
- Fix Edge Memory Issues: Old versions of Next.js may cause memory issues in edge runtime. You can fix this by upgrading to the latest version of Next.js (v14.1.3 or above).