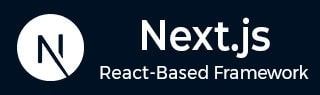
- Next.js - Home
- Next.js - Overview
- Next.js - Project Setup
- Next.js - Folder Structure
- Next.js - App Router
- Next.js - Page Router
- Next.js Features
- Next.js - Pages
- Next.js - Data Fetching
- Next.js - ISR
- Next.js - Static File Serving
- Next.js - Pre-Rendering
- Next.js - Partial Pre Rendering
- Next.js - Server Side Rendering
- Next.js - Client Side Rendering
- Next.js Routing
- Next.js - Routing
- Next.js - Nested Routing
- Next.js - Dynamic Routing
- Next.js - Parallel Routing
- Next.js - Imperative Routing
- Next.js - Shallow Routing
- Next.js - Intercepting Routes
- Next.js - Redirecting Routes
- Next.js - Navigation and Linking
- Next.js Configuration
- Next.js - TypeScript
- Next.js - Environment Variables
- Next.js - File Conventions
- Next.js - ESLint
- Next.js API & Backend
- Next.js - API Routes
- Next.js - Dynamic API Routes
- Next.js - Route Handlers
- Next.js - API MiddleWares
- Next.js - Response Helpers
- Next.js API Reference
- Next.js - CLI Commands
- Next.js - Functions
- Next.js - Directives
- Next.js - Components
- Next.js - Image Component
- Next.js - Font Component
- Next.js - Head Component
- Next.js - Form Component
- Next.js - Link Component
- Next.js - Script Component
- Next.js Styling & SEO
- Next.js - CSS Support
- Next.js - Global CSS Support
- Next.js - Meta Data
- Next.js Advanced Topics
- Next.js - Error Handling
- Next.js - Server Actions
- Next.js - Fast Refresh
- Next.js - Internationalization
- Next.js - Authentication
- Next.js - Session Management
- Next.js - Authorization
- Next.js - Caching
- Next.js - Data Caching
- Next.js - Router Caching
- Next.js - Full Route Caching
- Next.js - Request Memoization
- Next.js Performance Optimization
- Next.js - Optimizations
- Next.js - Image Optimization
- Next.js - Lazy Loading
- Next.js - Font Optimization
- Next.js - Video Optimization
- Next.js - Script Optimization
- Next.js - Memory Optimization
- Next.js - Using OpenTelemetry
- Next.js - Package Bundling Optimization
- Next.js Testing
- Next.js - Testing
- Next.js - Testing with Jest
- Next.js - Testing with Cypress
- Next.js - Testing with Vitest
- Next.js - Testing with Playwright
- Next.js Debugging & Deployment
- Next.js - Debugging
- Next.js - Deployment
- Next.js Useful Resources
- Next.js - Interview Questions
- Next.js - Quick Guide
- Next.js - Useful Resources
- Next.js - Discussion
Next.js - TypeScript Support
In this chapter, we will learn what is TypeScript, how to use TypeScript in Next.js, and example of using TypeScript in Next.js.
What is TypeScript?
TypeScript is a typed superset of JavaScript that compiles code into the plain JavaScript. It is a free and open-source high-level programming language. The main difference between JavaScript and TypeScript is that, JavaScript is a dynamic language that is used for small projects, while TypeScript is a static language that is used for large projects. If you want to learn more on TypeScript, visit our TypeScript Tutorial.
TypeScript Support in Next.js
Next.js comes with built-in TypeScript support. When you create a new Next.js project, you will be asked to include TypeScript in your project. Choosing to include typescript will automatically installing the necessary packages and configuration for TypeScript support. To add TypeScript to an existing project, rename a file to .ts or .tsx. Then run 'next dev' and 'next build' to automatically install the necessary dependencies and add a tsconfig.json file with the recommended config options.
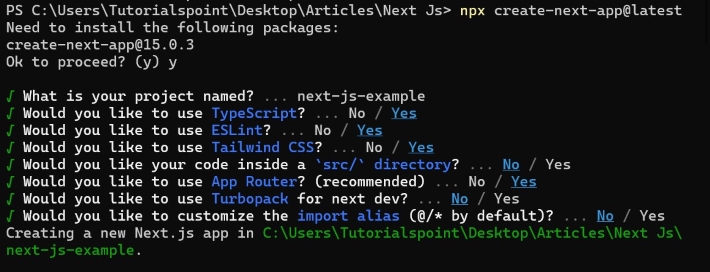
The above images shows setting up Next.js using create-next-app command. You can see that it asks to include TypeScript in the project.
Type Checking next.config.ts
In your Next.js configuration file, you can use TypeScript instead of JavaScript to import types and set configuration options. Consider the following code of configuration file in JavaScript and TypeScript.
// File - next.config.js (JavaScript) @type {import('next').NextConfig} const nextConfig = { /* config options here */ } module.exports = nextConfig // File - next.config.ts (TypeScript) import type { NextConfig } from 'next' const nextConfig: NextConfig = { /* config options here */ } export default nextConfig
The both of configuration achieve same setup. The TypeScript version uses explicit type imports and export default, with type checking at compile time. While, the JavaScript version uses JSDoc type annotations and CommonJS exports, with type checking happens via editor tooling.
Disabling TypeScript Errors in Production
By default, Next.js will cancel the production build if there are any errors in TypeScript. This is to prevent runtime errors in production. If you want to disable this behavior, you can set the ignoreBuildErrors option in your next.config.js file. If disabled, be sure you are running type checks as part of your build or deploy process, otherwise this can be very dangerous.
// File - next.config.js import type { NextConfig } from 'next' const nextConfig: NextConfig = { typescript: { ignoreBuildErrors: true, }, } export default nextConfig
Using Typescript Interfaces in Next.js
Interfaces are a TypeScript feature used to define the structure of an object. JavaScript does not have built-in support for interfaces. In Next.js, you can use interfaces to define the structure of props and state in components. Here is an example of using interfaces in Next.js.
// File - /app/api/users/[id]/route.ts import { NextRequest, NextResponse } from 'next/server' // Define User interface interface User { id: number name: string email: string } export async function GET( request: NextRequest, { params }: { params: { id: string } } ) { try { // Mock user data const user: User = { id: Number(params.id), name: "John Doe", email: "[email protected]" } return NextResponse.json(user) } catch (error) { return NextResponse.json( { message: 'User not found' }, { status: 400 } ) } }
Output
When you run the above code, you will get the following output:
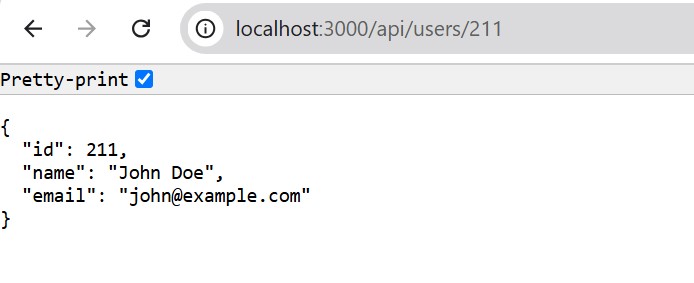