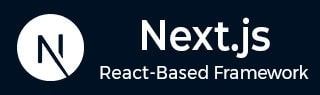
- Next.js - Home
- Next.js - Overview
- Next.js - Project Setup
- Next.js - Folder Structure
- Next.js - App Router
- Next.js - Page Router
- Next.js Features
- Next.js - Pages
- Next.js - Data Fetching
- Next.js - ISR
- Next.js - Static File Serving
- Next.js - Pre-Rendering
- Next.js - Partial Pre Rendering
- Next.js - Server Side Rendering
- Next.js - Client Side Rendering
- Next.js Routing
- Next.js - Routing
- Next.js - Nested Routing
- Next.js - Dynamic Routing
- Next.js - Parallel Routing
- Next.js - Imperative Routing
- Next.js - Shallow Routing
- Next.js - Intercepting Routes
- Next.js - Redirecting Routes
- Next.js - Navigation and Linking
- Next.js Configuration
- Next.js - TypeScript
- Next.js - Environment Variables
- Next.js - File Conventions
- Next.js - ESLint
- Next.js API & Backend
- Next.js - API Routes
- Next.js - Dynamic API Routes
- Next.js - Route Handlers
- Next.js - API MiddleWares
- Next.js - Response Helpers
- Next.js API Reference
- Next.js - CLI Commands
- Next.js - Functions
- Next.js - Directives
- Next.js - Components
- Next.js - Image Component
- Next.js - Font Component
- Next.js - Head Component
- Next.js - Form Component
- Next.js - Link Component
- Next.js - Script Component
- Next.js Styling & SEO
- Next.js - CSS Support
- Next.js - Global CSS Support
- Next.js - Meta Data
- Next.js Advanced Topics
- Next.js - Error Handling
- Next.js - Server Actions
- Next.js - Fast Refresh
- Next.js - Internationalization
- Next.js - Authentication
- Next.js - Session Management
- Next.js - Authorization
- Next.js - Caching
- Next.js - Data Caching
- Next.js - Router Caching
- Next.js - Full Route Caching
- Next.js - Request Memoization
- Next.js Performance Optimization
- Next.js - Optimizations
- Next.js - Image Optimization
- Next.js - Lazy Loading
- Next.js - Font Optimization
- Next.js - Video Optimization
- Next.js - Script Optimization
- Next.js - Memory Optimization
- Next.js - Using OpenTelemetry
- Next.js - Package Bundling Optimization
- Next.js Testing
- Next.js - Testing
- Next.js - Testing with Jest
- Next.js - Testing with Cypress
- Next.js - Testing with Vitest
- Next.js - Testing with Playwright
- Next.js Debugging & Deployment
- Next.js - Debugging
- Next.js - Deployment
- Next.js Useful Resources
- Next.js - Interview Questions
- Next.js - Quick Guide
- Next.js - Useful Resources
- Next.js - Discussion
Next.js - Functions
Function Reference
Next.js have several builtin APIs, functions, hooks and methods to handle data at client side and server side. This chapter will give reference to all of those APIs, functions, hooks and methods in Next.js with some added examples.
Page Router Functions
Following table provide reference to all page router functions in Next.js
Functions | Explanation |
---|---|
useRouter() | The useRouter function is a Next.js hook that helps to access `router` object inside any component in the application. |
useAmp() | The useAmp hook in Next.js is a utility that allows you to detect if the current page is being rendered as an AMP (Accelerated Mobile Pages) page |
getStaticProps() | The getStaticProps is a data-fetching method that allows you to fetch data at build time. This method is used in static site generation. |
getStaticPaths() | The getStaticPaths is a data-fetching method used with getStaticProps to generate dynamic routes at build time. |
getServerSideProps() | The getServerSideProps is a data-fetching method that is used to fetch data at request time on the server |
userAgent() | This extends web request API with additional properties and methods to interact with user agent object from the request. |
useReportWebVitals() | The useReportWebVitals hook is used with your analytics service to report Core Web Vitals. |
NextResponse() | NextResponse extends the Web Response API with additional convenience methods. |
NextRequest() | NextRequest extends the Web Request API with additional convenience methods. |
getInitialProps() | The getInitialProps is a data-fetching method in Next.js that is used to fetch data both on the server and client side. |
App Router Functions
Following table provide reference to all function used in Next.js App Router.
Functions | Explanation |
---|---|
after() | The after function is used to schedule a code to execute after pre rendering the page. |
cacheLife() | Specifies the lifetime of cached data to optimize performance and reduce network requests. |
cacheTag() | Enables tagging of cache entries for targeted invalidation or management. |
cookies() | Allows reading, setting, or manipulating cookies in Next.js applications. |
draftMode() | Enables draft mode for previewing unpublished content in a Next.js application. |
fetch() | Provides a wrapper around the native fetch API with enhanced features for data fetching in Next.js. |
forbidden() | Returns a 403 HTTP status code when access to a resource is forbidden. |
generateMetadata() | Allows generating metadata for a page, including SEO-related properties. |
generateImageMetadata() | Generates metadata for images, such as dimensions or alternative text, for optimized rendering. |
generateSitemaps() | Automatically generates XML sitemaps for search engine indexing in Next.js applications. |
generateStaticParams() | Generates parameters for dynamic routes during static site generation. |
generateViewport() | Generates viewport meta tags dynamically for responsive design. |
headers() | Accesses or manipulates HTTP headers for requests and responses in Next.js. |
ImageResponse() | Used to generate and serve dynamic images in response to requests in Next.js. |
NextRequest() | Extends the Web Request API with additional convenience methods. |
NextResponse() | Extends the Web Response API with additional convenience methods. |
notFound() | Returns a 404 HTTP status code when a resource is not found. |
permanentRedirect() | Creates a 301 HTTP status code for permanent URL redirection. |
redirect() | Generates a redirection response with customizable HTTP status codes. |
revalidatePath() | Revalidates a specific path to fetch the latest data and update the cache. |
revalidateTag() | Triggers revalidation for resources associated with a specific tag. |
unauthorized() | Returns a 401 HTTP status code when authentication is required but missing or invalid. |
unstable_cache() | Provides experimental cache functionality for advanced caching scenarios. |
unstable_noStore() | Prevents caching of data, forcing a fresh fetch on every request. |
unstable_rethrow() | Re-throws errors encountered during rendering or fetching for debugging purposes. |
useParams() | Retrieves dynamic route parameters in a client component. |
usePathname() | Provides the current pathname of the application in a client component. |
useReportWebVitals() | Reports Core Web Vitals to your analytics service. |
useSearchParams() | Provides access to URL query parameters in a client component. |
useSelectedLayoutSegment() | Returns the currently selected segment in a layout-based routing setup. |
userAgent() | Provides access to the user agent string for client-side rendering and feature detection. |
Next.js Functions Examples
Example 1
Let's look at an example to understand how to use generateMetadata() function The generateMetadata() function is used to dynamically generate metadata such as titles, description of a webpage based on route parameters or fetched data.
In the following code, we will generate a dynamic title (ie, the metadata) for a component based on page selected by user.
// app/products/[id]/page.tsx import { Metadata } from "next"; type Props ={ params: { id: string; }; } // Dynamic Metadata function export const generateMetadata = ({ params }: Props): Metadata => { return { title: `Product ${params.id} - My Store`, }; } export default function ProductPage({params }: Props) { return ( <div> <h1>Product {params.id}</h1> <p>This is the product page for item {params.id}</p> </div> ); }
Output
In the output, you can see that the title of page is changing based on route we choose.
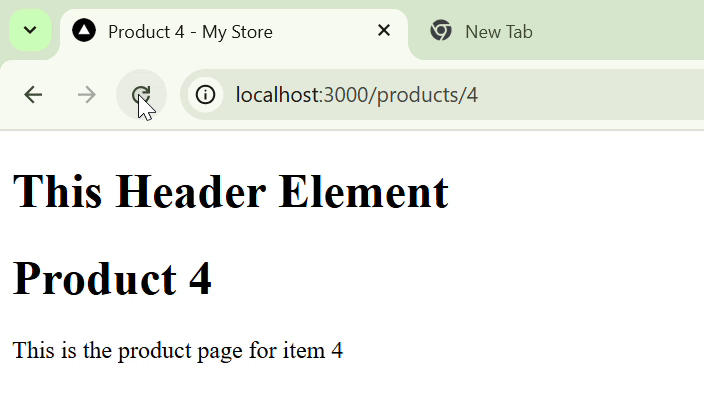
Example 2
Now, let's look at the useAmp method. The useAmp method is a utility method used to detect if the current page is being rendered as an AMP (Accelerated Mobile Pages) page.
The code below shows how to use the useAmp method in Next.js. We created a button that toggles between AMP version and Non Amp version of same page. See the output below.
// pages/index.tsx import { useAmp } from 'next/amp'; export const config = { amp: 'hybrid' }; // Enable Hybrid AMP mode export default function Home() { const isAmp = useAmp(); return ( <div> <h1>Welcome to Next.js with AMP</h1> {isAmp ? ( <p>You are viewing the AMP version of this page.</p> ) : ( <p>You are viewing the standard version of this page.</p> )} <a href={isAmp ? '/' : '/?amp=1'}> Switch to {isAmp ? 'Standard' : 'AMP'} Mode </a> </div> ); }
Output
In the output, AMP mode is getting toggled on clicking the link.
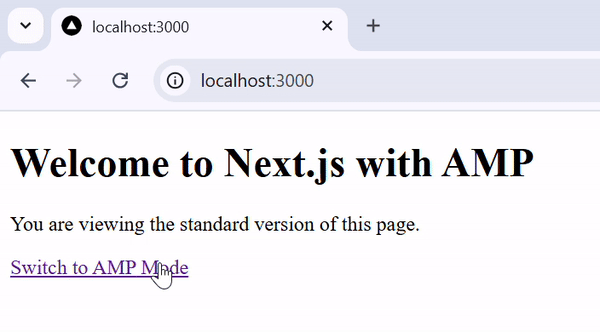