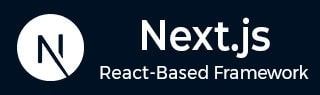
- Next.js - Home
- Next.js - Overview
- Next.js - Project Setup
- Next.js - Folder Structure
- Next.js - App Router
- Next.js - Page Router
- Next.js Features
- Next.js - Pages
- Next.js - Data Fetching
- Next.js - ISR
- Next.js - Static File Serving
- Next.js - Pre-Rendering
- Next.js - Partial Pre Rendering
- Next.js - Server Side Rendering
- Next.js - Client Side Rendering
- Next.js Routing
- Next.js - Routing
- Next.js - Nested Routing
- Next.js - Dynamic Routing
- Next.js - Parallel Routing
- Next.js - Imperative Routing
- Next.js - Shallow Routing
- Next.js - Intercepting Routes
- Next.js - Redirecting Routes
- Next.js - Navigation and Linking
- Next.js Configuration
- Next.js - TypeScript
- Next.js - Environment Variables
- Next.js - File Conventions
- Next.js - ESLint
- Next.js API & Backend
- Next.js - API Routes
- Next.js - Dynamic API Routes
- Next.js - Route Handlers
- Next.js - API MiddleWares
- Next.js - Response Helpers
- Next.js API Reference
- Next.js - CLI Commands
- Next.js - Functions
- Next.js - Directives
- Next.js - Components
- Next.js - Image Component
- Next.js - Font Component
- Next.js - Head Component
- Next.js - Form Component
- Next.js - Link Component
- Next.js - Script Component
- Next.js Styling & SEO
- Next.js - CSS Support
- Next.js - Global CSS Support
- Next.js - Meta Data
- Next.js Advanced Topics
- Next.js - Error Handling
- Next.js - Server Actions
- Next.js - Fast Refresh
- Next.js - Internationalization
- Next.js - Authentication
- Next.js - Session Management
- Next.js - Authorization
- Next.js - Caching
- Next.js - Data Caching
- Next.js - Router Caching
- Next.js - Full Route Caching
- Next.js - Request Memoization
- Next.js Performance Optimization
- Next.js - Optimizations
- Next.js - Image Optimization
- Next.js - Lazy Loading
- Next.js - Font Optimization
- Next.js - Video Optimization
- Next.js - Script Optimization
- Next.js - Memory Optimization
- Next.js - Using OpenTelemetry
- Next.js - Package Bundling Optimization
- Next.js Testing
- Next.js - Testing
- Next.js - Testing with Jest
- Next.js - Testing with Cypress
- Next.js - Testing with Vitest
- Next.js - Testing with Playwright
- Next.js Debugging & Deployment
- Next.js - Debugging
- Next.js - Deployment
- Next.js Useful Resources
- Next.js - Interview Questions
- Next.js - Quick Guide
- Next.js - Useful Resources
- Next.js - Discussion
Next.js - Data Fetching
Data Fetching
Next.js comes with all the data fetching capabilities of React.js. It supports multiple data-fetching methods, such as static generation (SSG), server-side rendering (SSR), and client-side fetching. Next.js ensure security, by fetching data on server-side and then releasing it to the client-side on request. The server-side data fetching provide better performance and SEO results by reducing client-side javascript code
Types of Data Fetching
Next.js offers three types of data fetching methods:
Server-side Fetching
In server-side fetching, the data is fetched on the server-side and then released to the client-side. It is two types.
- Static Site Generation
- Server-side Rendering
Static Site Generation
The static generation is the default behavior in Next.js. In this method, Next.js generates these pages at build time and reuses them for every request. The static generation is the recommended method for pages that do not require frequently updated data.
In the following example, we implement a static page, which will fetch data from an API and display it on the page. We use the fetch function to make a request to the API and then use the json
method to parse the response as JSON.
export async function getStaticProps() { const res = await fetch('https://jsonplaceholder.typicode.com/posts/1'); const post = await res.json(); return { props: { post }, }; } export default function Post({ post }) { return ( <div> <h1>{post.title}</h1> <p>{post.body}</p> </div> ); }
Server-side Rendering
In server-side rendering, the data will be fetched for every request to server. This method is recommended for pages that require frequently updated data.
In the example below, we used the getServerSideProps function to fetch data from an API and pass it to the page as props. This ensures that the user will see the latest data on every request.
export async function getServerSideProps() { const res = await fetch('https://jsonplaceholder.typicode.com/posts/1'); const post = await res.json(); return { props: { post }, }; } export default function Post({ post }) { return ( <div> <h1>{post.title}</h1> <p>{post.body}</p> </div> ); }
Client-side Fetching
In client-side fetching, the data fetching will happen on user's browser. This is not a recommended approach as it affect performance and SEO results of website.
The example below uses, the react useEffect hook to fetch data from an API and set it to the state. The data is then used to render the page.
"use client"; import { useEffect, useState } from 'react'; export default function Post() { const [post, setPost] = useState(null); useEffect(() => { fetch('https://jsonplaceholder.typicode.com/posts/1') .then(res => res.json()) .then(data => setPost(data)); }, []); if (!post) return <p>Loading...</p>; return ( <div> <h1>{post.title}</h1> <p>{post.body}</p> </div> ); }
Incremental Static Regeneration
Incremental Static Regeneration is a feature in Next.js by which the static pages can be updated after deployment without requiring a full rebuild. This means that pages can be statically generated at build time and then incrementally updated as needed while the application is running.
In the following example, we will create a page that fetches data from an API and displays it on the page. Here we set revalidate: 10
, this ensures that the page updates at most every 10 seconds when a new request comes in.
// File: pages/index.js import { useEffect } from 'react'; import { getServerSideProps } from 'next'; export async function getStaticProps() { const data = await fetchData(); // Fetch data from API return { props: { data }, revalidate: 10, // Regenerate the page every 10 seconds }; } export default function Home({ data }) { return ( <div> <h1>Home Page</h1> <p>This is the home page.</p> <div>{JSON.stringify(data)}</div> </div> ); }