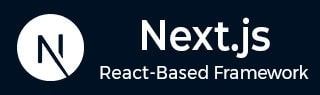
- Next.js - Home
- Next.js - Overview
- Next.js - Project Setup
- Next.js - Folder Structure
- Next.js - App Router
- Next.js - Page Router
- Next.js Features
- Next.js - Pages
- Next.js - Data Fetching
- Next.js - ISR
- Next.js - Static File Serving
- Next.js - Pre-Rendering
- Next.js - Partial Pre Rendering
- Next.js - Server Side Rendering
- Next.js - Client Side Rendering
- Next.js Routing
- Next.js - Routing
- Next.js - Nested Routing
- Next.js - Dynamic Routing
- Next.js - Parallel Routing
- Next.js - Imperative Routing
- Next.js - Shallow Routing
- Next.js - Intercepting Routes
- Next.js - Redirecting Routes
- Next.js - Navigation and Linking
- Next.js Configuration
- Next.js - TypeScript
- Next.js - Environment Variables
- Next.js - File Conventions
- Next.js - ESLint
- Next.js API & Backend
- Next.js - API Routes
- Next.js - Dynamic API Routes
- Next.js - Route Handlers
- Next.js - API MiddleWares
- Next.js - Response Helpers
- Next.js API Reference
- Next.js - CLI Commands
- Next.js - Functions
- Next.js - Directives
- Next.js - Components
- Next.js - Image Component
- Next.js - Font Component
- Next.js - Head Component
- Next.js - Form Component
- Next.js - Link Component
- Next.js - Script Component
- Next.js Styling & SEO
- Next.js - CSS Support
- Next.js - Global CSS Support
- Next.js - Meta Data
- Next.js Advanced Topics
- Next.js - Error Handling
- Next.js - Server Actions
- Next.js - Fast Refresh
- Next.js - Internationalization
- Next.js - Authentication
- Next.js - Session Management
- Next.js - Authorization
- Next.js - Caching
- Next.js - Data Caching
- Next.js - Router Caching
- Next.js - Full Route Caching
- Next.js - Request Memoization
- Next.js Performance Optimization
- Next.js - Optimizations
- Next.js - Image Optimization
- Next.js - Lazy Loading
- Next.js - Font Optimization
- Next.js - Video Optimization
- Next.js - Script Optimization
- Next.js - Memory Optimization
- Next.js - Using OpenTelemetry
- Next.js - Package Bundling Optimization
- Next.js Testing
- Next.js - Testing
- Next.js - Testing with Jest
- Next.js - Testing with Cypress
- Next.js - Testing with Vitest
- Next.js - Testing with Playwright
- Next.js Debugging & Deployment
- Next.js - Debugging
- Next.js - Deployment
- Next.js Useful Resources
- Next.js - Interview Questions
- Next.js - Quick Guide
- Next.js - Useful Resources
- Next.js - Discussion
Next.js - after() Function
The after() Function
The Next.js after()
function is used to schedule a function to execute after pre rendering the page or after sending the response to the client. This function is helpful for performing cleanup tasks, logging, or any other post-processing operations.
Syntax
Following is the syntax of after() function:
import { after } from 'next/server' export async function after(response: Response, request: NextRequest, event: NextFetchEvent) { // Modify the response if needed const newResponse = new Response(response.body, response) return newResponse; }
Parameters
The after()
function takes three parameters:
- response: The response object that will be sent to the client
- request: The NextRequest object representing the incoming request
- event: The NextFetchEvent object representing the fetch event
Return Value
The after()
function will return a Response object. This can be the original response object or a new response object with modifications.
Key Points
- The after() function must be exported from middleware of Next.js application
- It is executed after the response is sent to the client
- You can use the after() function to perform cleanup tasks, logging, or modify the response
Example 1
In the code below, we demonstrate how to use the after() function to log errors and add a custom header to the response in a Next.js application.
// app/api/hello/route.ts import { NextRequest, NextResponse, after } from 'next/server' export async function GET(request: NextRequest) { return NextResponse.json({ message: 'Hello, World!' }) } export async function after(response: Response, request: NextRequest, event: NextFetchEvent) { if (response.status === 500) { console.error(`Error in request to ${request.nextUrl.pathname}`) } const newResponse = new Response(response.body, response) newResponse.headers.set('x-response-time', Date.now().toString()) return newResponse; }
Example 2
In the code below, we demonstrate how to use the after() function to log errors and modify the response in a Next.js application.
// app/api/hello/route.ts import { NextRequest, NextResponse, after } from 'next/server' export async function GET(request: NextRequest) { return NextResponse.json({ message: 'Hello, World!' }) } export async function after(response: Response, request: NextRequest, event: NextFetchEvent) { if (response.status === 500) { console.error(`Error in request to ${request.nextUrl.pathname}`) } const newResponse = new Response(response.body, response) newResponse.headers.set('x-response-time', Date.now().toString()) return newResponse; }
Advertisements