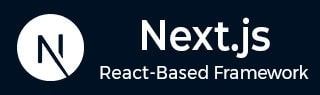
- Next.js - Home
- Next.js - Overview
- Next.js - Project Setup
- Next.js - Folder Structure
- Next.js - App Router
- Next.js - Page Router
- Next.js Features
- Next.js - Pages
- Next.js - Data Fetching
- Next.js - ISR
- Next.js - Static File Serving
- Next.js - Pre-Rendering
- Next.js - Partial Pre Rendering
- Next.js - Server Side Rendering
- Next.js - Client Side Rendering
- Next.js Routing
- Next.js - Routing
- Next.js - Nested Routing
- Next.js - Dynamic Routing
- Next.js - Parallel Routing
- Next.js - Imperative Routing
- Next.js - Shallow Routing
- Next.js - Intercepting Routes
- Next.js - Redirecting Routes
- Next.js - Navigation and Linking
- Next.js Configuration
- Next.js - TypeScript
- Next.js - Environment Variables
- Next.js - File Conventions
- Next.js - ESLint
- Next.js API & Backend
- Next.js - API Routes
- Next.js - Dynamic API Routes
- Next.js - Route Handlers
- Next.js - API MiddleWares
- Next.js - Response Helpers
- Next.js API Reference
- Next.js - CLI Commands
- Next.js - Functions
- Next.js - Directives
- Next.js - Components
- Next.js - Image Component
- Next.js - Font Component
- Next.js - Head Component
- Next.js - Form Component
- Next.js - Link Component
- Next.js - Script Component
- Next.js Styling & SEO
- Next.js - CSS Support
- Next.js - Global CSS Support
- Next.js - Meta Data
- Next.js Advanced Topics
- Next.js - Error Handling
- Next.js - Server Actions
- Next.js - Fast Refresh
- Next.js - Internationalization
- Next.js - Authentication
- Next.js - Session Management
- Next.js - Authorization
- Next.js - Caching
- Next.js - Data Caching
- Next.js - Router Caching
- Next.js - Full Route Caching
- Next.js - Request Memoization
- Next.js Performance Optimization
- Next.js - Optimizations
- Next.js - Image Optimization
- Next.js - Lazy Loading
- Next.js - Font Optimization
- Next.js - Video Optimization
- Next.js - Script Optimization
- Next.js - Memory Optimization
- Next.js - Using OpenTelemetry
- Next.js - Package Bundling Optimization
- Next.js Testing
- Next.js - Testing
- Next.js - Testing with Jest
- Next.js - Testing with Cypress
- Next.js - Testing with Vitest
- Next.js - Testing with Playwright
- Next.js Debugging & Deployment
- Next.js - Debugging
- Next.js - Deployment
- Next.js Useful Resources
- Next.js - Interview Questions
- Next.js - Quick Guide
- Next.js - Useful Resources
- Next.js - Discussion
Next.js - getStaticProps Function
In Next.js getStaticProps() function is used fetch API data at the build time to the server. In this chapter we will learn, what is getStaticProps function, how to use it, syntax and example usage of getStaticProps method.
Next.js getStaticProps Function
The getStaticProps function in Next.js is a data-fetching method used for fetching data from sources at build time for pages that are pre-rendered as HTML. This data fetching happens once at build time, and same page is severed to every users. The data can be updated only with rebuild of the application, hence this method is not suitable for pages that changes regularly.
Syntax
Following is syntax of getStaticProps function.
export async function getStaticProps() { // Simulate fetching data from an API const response = await fetch('https://link/to/api'); const data = await response.json(); return { props: { data, // Return data to the component }, }; }
Example of Using getStaticPaths in Next.js
In the below example code, we fetched data from an external API using 'getStaticProps()' function. This function will fetch data once at build time, and then create a complete HTML file based on it. Since we are running application in development mode, HTML file will created at development environment itself. See the example below.
// page/index.tsx import React from 'react'; // Export getStaticProps function to fetch data export async function getStaticProps() { // Simulate fetching data from an API const response = await fetch('https://link/to/api'); const posts = await response.json(); return { props: { posts, // This will be passed to Blog component as props }, }; } // Receive posts prop from getStaticProps function export default function Blog({ posts }) { return ( <div> <h1>Blog Posts</h1> <ul> {posts.map((post) => ( <li key={post.id}>{post.title}</li> ))} </ul> </div> ); }
Output
In this output, we are displaying data from an API in static page.
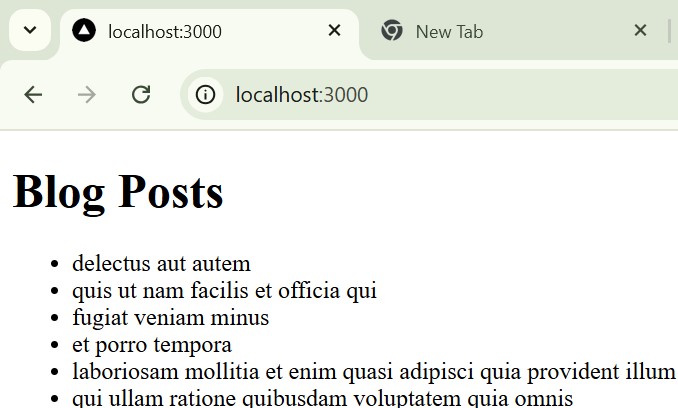