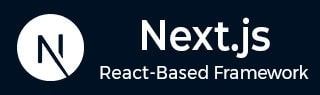
- Next.js - Home
- Next.js - Overview
- Next.js - Project Setup
- Next.js - Folder Structure
- Next.js - App Router
- Next.js - Page Router
- Next.js Features
- Next.js - Pages
- Next.js - Data Fetching
- Next.js - ISR
- Next.js - Static File Serving
- Next.js - Pre-Rendering
- Next.js - Partial Pre Rendering
- Next.js - Server Side Rendering
- Next.js - Client Side Rendering
- Next.js Routing
- Next.js - Routing
- Next.js - Nested Routing
- Next.js - Dynamic Routing
- Next.js - Parallel Routing
- Next.js - Imperative Routing
- Next.js - Shallow Routing
- Next.js - Intercepting Routes
- Next.js - Redirecting Routes
- Next.js - Navigation and Linking
- Next.js Configuration
- Next.js - TypeScript
- Next.js - Environment Variables
- Next.js - File Conventions
- Next.js - ESLint
- Next.js API & Backend
- Next.js - API Routes
- Next.js - Dynamic API Routes
- Next.js - Route Handlers
- Next.js - API MiddleWares
- Next.js - Response Helpers
- Next.js API Reference
- Next.js - CLI Commands
- Next.js - Functions
- Next.js - Directives
- Next.js - Components
- Next.js - Image Component
- Next.js - Font Component
- Next.js - Head Component
- Next.js - Form Component
- Next.js - Link Component
- Next.js - Script Component
- Next.js Styling & SEO
- Next.js - CSS Support
- Next.js - Global CSS Support
- Next.js - Meta Data
- Next.js Advanced Topics
- Next.js - Error Handling
- Next.js - Server Actions
- Next.js - Fast Refresh
- Next.js - Internationalization
- Next.js - Authentication
- Next.js - Session Management
- Next.js - Authorization
- Next.js - Caching
- Next.js - Data Caching
- Next.js - Router Caching
- Next.js - Full Route Caching
- Next.js - Request Memoization
- Next.js Performance Optimization
- Next.js - Optimizations
- Next.js - Image Optimization
- Next.js - Lazy Loading
- Next.js - Font Optimization
- Next.js - Video Optimization
- Next.js - Script Optimization
- Next.js - Memory Optimization
- Next.js - Using OpenTelemetry
- Next.js - Package Bundling Optimization
- Next.js Testing
- Next.js - Testing
- Next.js - Testing with Jest
- Next.js - Testing with Cypress
- Next.js - Testing with Vitest
- Next.js - Testing with Playwright
- Next.js Debugging & Deployment
- Next.js - Debugging
- Next.js - Deployment
- Next.js Useful Resources
- Next.js - Interview Questions
- Next.js - Quick Guide
- Next.js - Useful Resources
- Next.js - Discussion
Next.js - notFound() Function
The notFound() Function
The Next.js notFound()
function is used to render the not-found file within a route segment. This function is helpful when you want to display a custom 404 page for a specific route or segment of your application.
Syntax
Following is the syntax of notFound()
function:
import { notFound } from 'next/navigation' export default async function Profile({ params }) { const user = await fetchUser((await params).id) // If user not found, render the not-found page if (!user) { notFound() } }
Parameters
The notFound()
function does not take any parameters.
Return Value
The notFound()
function does not return any value. The notFound does not require you to use 'return notFound()' as it uses the TypeScript never type.
Key Points
- The notFound() function is used to render the not-found file within a route segment.
- Invoking the notFound() function throws a NEXT_NOT_FOUND error and terminates rendering of the route segment in which it was thrown.
- You can create a not-found.tsx file in the root directory of your application. This file defines the UI shown when notFound() is called.
Example
In the example below, we are creating a not-found.tsx file in the root directory of our application. This file defines the UI shown when notFound() is called.
// app/not-found.tsx export default function Custom404() { return ( <div> <h1>404 - Page Not Found</h1> <p>Sorry, the page you are looking for does not exist.</p> </div> ); } // app/posts/[id]/page.tsx import { notFound } from 'next/navigation'; async function getPost(id: string) { const post = await fetch(`/api/posts/${id}`); if (!post.ok) return null; return post.json(); } export default async function Post({ params }: { params: { id: string } }) { const post = await getPost(params.id); if (!post) { notFound(); // This triggers the not-found.tsx page } return ( <article> <h1>{post.title}</h1> <p>{post.content}</p> </article> ); }
Output
In the output, when we try to visit any random pages, 404 error which we defined is displayed.
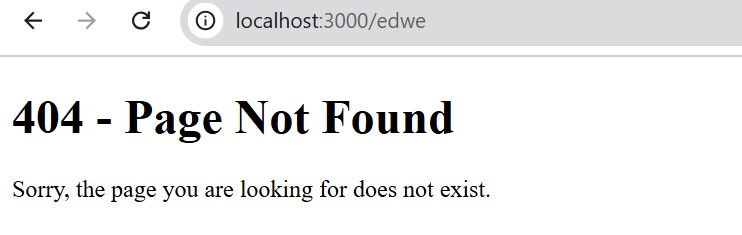
Advertisements