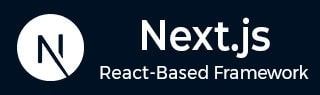
- Next.js - Home
- Next.js - Overview
- Next.js - Project Setup
- Next.js - Folder Structure
- Next.js - App Router
- Next.js - Page Router
- Next.js Features
- Next.js - Pages
- Next.js - Data Fetching
- Next.js - ISR
- Next.js - Static File Serving
- Next.js - Pre-Rendering
- Next.js - Partial Pre Rendering
- Next.js - Server Side Rendering
- Next.js - Client Side Rendering
- Next.js Routing
- Next.js - Routing
- Next.js - Nested Routing
- Next.js - Dynamic Routing
- Next.js - Parallel Routing
- Next.js - Imperative Routing
- Next.js - Shallow Routing
- Next.js - Intercepting Routes
- Next.js - Redirecting Routes
- Next.js - Navigation and Linking
- Next.js Configuration
- Next.js - TypeScript
- Next.js - Environment Variables
- Next.js - File Conventions
- Next.js - ESLint
- Next.js API & Backend
- Next.js - API Routes
- Next.js - Dynamic API Routes
- Next.js - Route Handlers
- Next.js - API MiddleWares
- Next.js - Response Helpers
- Next.js API Reference
- Next.js - CLI Commands
- Next.js - Functions
- Next.js - Directives
- Next.js - Components
- Next.js - Image Component
- Next.js - Font Component
- Next.js - Head Component
- Next.js - Form Component
- Next.js - Link Component
- Next.js - Script Component
- Next.js Styling & SEO
- Next.js - CSS Support
- Next.js - Global CSS Support
- Next.js - Meta Data
- Next.js Advanced Topics
- Next.js - Error Handling
- Next.js - Server Actions
- Next.js - Fast Refresh
- Next.js - Internationalization
- Next.js - Authentication
- Next.js - Session Management
- Next.js - Authorization
- Next.js - Caching
- Next.js - Data Caching
- Next.js - Router Caching
- Next.js - Full Route Caching
- Next.js - Request Memoization
- Next.js Performance Optimization
- Next.js - Optimizations
- Next.js - Image Optimization
- Next.js - Lazy Loading
- Next.js - Font Optimization
- Next.js - Video Optimization
- Next.js - Script Optimization
- Next.js - Memory Optimization
- Next.js - Using OpenTelemetry
- Next.js - Package Bundling Optimization
- Next.js Testing
- Next.js - Testing
- Next.js - Testing with Jest
- Next.js - Testing with Cypress
- Next.js - Testing with Vitest
- Next.js - Testing with Playwright
- Next.js Debugging & Deployment
- Next.js - Debugging
- Next.js - Deployment
- Next.js Useful Resources
- Next.js - Interview Questions
- Next.js - Quick Guide
- Next.js - Useful Resources
- Next.js - Discussion
Next.js - permanentRedirect() Function
The permanentRedirect() Function
The Next.js permanentRedirect()
function is used to redirect the client to a new URL with a permanent redirect status code (301). This function is helpful when you want to permanently redirect a user to a new location.
Syntax
Following is the syntax of permanentRedirect()
function:
import { permanentRedirect } from 'next/navigation' export default async function Profile({ params }) { const user = await fetchUser((await params).id) // If user not found, redirect to the home page if (!user) { permanentRedirect('/') } }
Parameters
The permanentRedirect()
function takes two parameters:
- path - string - The URL to redirect to. Can be a relative or absolute path.
- type - 'replace' (default) or 'push' (default in Server Actions) - The type of redirect to perform.
By default, permanentRedirect will use push (adding a new entry to the browser history stack) in Server Actions and replace (replacing the current URL in the browser history stack) everywhere else. You can override this behavior by specifying the type parameter.
Return Value
The permanentRedirect()
function does not return any value. It does not require you to use 'return permanentRedirect()' as it uses the TypeScript never type.
Example 1
In the example below, we are redirecting feature to the product page we created in previous chapter. On clicking the submit button, user will get a confirmation of the order and redirect them to home page.
"use client"; import { permanentRedirect } from 'next/navigation' import { PageProps } from "next/dist/shared/lib/router/utils/page-props"; export default function ProductPage({ params }: PageProps) { const handleClick = () =>{ alert("Order Placed..."); // Redirect to home page after placing order permanentRedirect('/') }; return ( <div> <h1>Product {params.id}</h1> <p>This is the product page for item {params.id}</p> <button onClick={handleClick}>Order Item</button> </div> ); }
Output
In the output, after placing order user is getting redirected to home page.
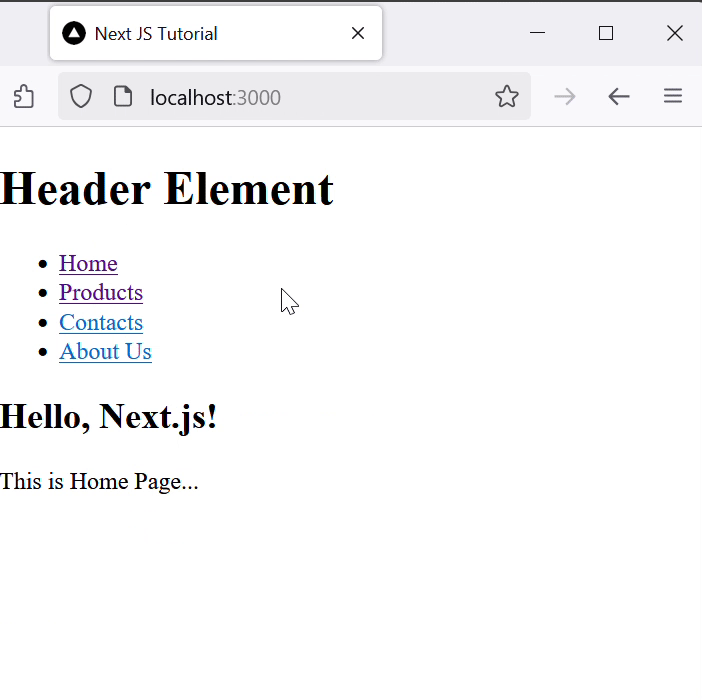
Example 2
Invoking the permanentRedirect() function throws a NEXT_REDIRECT error and terminates rendering of the route segment in which it was thrown. In the example below, we are fetching the team from the database and redirecting user to login page if team is not found.
import { permanentRedirect } from 'next/navigation' async function fetchTeam(id) { const res = await fetch('https://...') if (!res.ok) return undefined return res.json() } export default async function Profile({ params }) { const team = await fetchTeam((await params).id) if (!team) { permanentRedirect('/login') } }