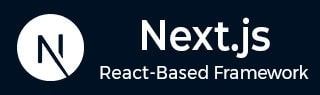
- Next.js - Home
- Next.js - Overview
- Next.js - Project Setup
- Next.js - Folder Structure
- Next.js - App Router
- Next.js - Page Router
- Next.js Features
- Next.js - Pages
- Next.js - Data Fetching
- Next.js - ISR
- Next.js - Static File Serving
- Next.js - Pre-Rendering
- Next.js - Partial Pre Rendering
- Next.js - Server Side Rendering
- Next.js - Client Side Rendering
- Next.js Routing
- Next.js - Routing
- Next.js - Nested Routing
- Next.js - Dynamic Routing
- Next.js - Parallel Routing
- Next.js - Imperative Routing
- Next.js - Shallow Routing
- Next.js - Intercepting Routes
- Next.js - Redirecting Routes
- Next.js - Navigation and Linking
- Next.js Configuration
- Next.js - TypeScript
- Next.js - Environment Variables
- Next.js - File Conventions
- Next.js - ESLint
- Next.js API & Backend
- Next.js - API Routes
- Next.js - Dynamic API Routes
- Next.js - Route Handlers
- Next.js - API MiddleWares
- Next.js - Response Helpers
- Next.js API Reference
- Next.js - CLI Commands
- Next.js - Functions
- Next.js - Directives
- Next.js - Components
- Next.js - Image Component
- Next.js - Font Component
- Next.js - Head Component
- Next.js - Form Component
- Next.js - Link Component
- Next.js - Script Component
- Next.js Styling & SEO
- Next.js - CSS Support
- Next.js - Global CSS Support
- Next.js - Meta Data
- Next.js Advanced Topics
- Next.js - Error Handling
- Next.js - Server Actions
- Next.js - Fast Refresh
- Next.js - Internationalization
- Next.js - Authentication
- Next.js - Session Management
- Next.js - Authorization
- Next.js - Caching
- Next.js - Data Caching
- Next.js - Router Caching
- Next.js - Full Route Caching
- Next.js - Request Memoization
- Next.js Performance Optimization
- Next.js - Optimizations
- Next.js - Image Optimization
- Next.js - Lazy Loading
- Next.js - Font Optimization
- Next.js - Video Optimization
- Next.js - Script Optimization
- Next.js - Memory Optimization
- Next.js - Using OpenTelemetry
- Next.js - Package Bundling Optimization
- Next.js Testing
- Next.js - Testing
- Next.js - Testing with Jest
- Next.js - Testing with Cypress
- Next.js - Testing with Vitest
- Next.js - Testing with Playwright
- Next.js Debugging & Deployment
- Next.js - Debugging
- Next.js - Deployment
- Next.js Useful Resources
- Next.js - Interview Questions
- Next.js - Quick Guide
- Next.js - Useful Resources
- Next.js - Discussion
Next.js - unauthorized() Function
The unauthorized() Function
The Next.js unauthorized()
function is used to throw an error that renders a Next.js 401 error page. It's useful for handling authorization errors in your application. You can customize the UI using the unauthorized.js file.
Syntax
The syntax for the unauthorized()
function is as follows:
import { verifySession } from '@/app/lib/dal' import { unauthorized } from 'next/navigation' export default async function DashboardPage() { const session = await verifySession() // if session is not verified, throw an error if (!session) { unauthorized() } }
Parameters
The unauthorized()
function accepts no parameters.
Return Value
The unauthorized()
function does not return any value.
Enable Unauthorized Function
The unauthorized()
function is an experimental feature in Next.js. To enable it, you need to set 'authInterrupts' configuration option in your next.config.js file.
import type { NextConfig } from 'next' const nextConfig: NextConfig = { experimental: { authInterrupts: true, }, } export default nextConfig
Example 1
You can use unauthorized function to display the unauthorized.js file with a login UI. The code below shows how to use the unauthorized() function to throw an error that renders the unauthorized.js file.
// app/dashboard/page.tsx import { verifySession } from '@/app/lib/dal' import { unauthorized } from 'next/navigation' export default async function DashboardPage() { const session = await verifySession() if (!session) { unauthorized() } return <div>Dashboard</div> } // app/unauthorized.js import Login from '@/app/components/Login' export default function UnauthorizedPage() { return ( <main> <h1>401 - Unauthorized</h1> <p>Please log in to access this page.</p> <Login /> </main> ) }
Output
The image below shows the output of the code snippet above. When we try to access the dashboard page without logging in, we get the unauthorized.js file with a login UI.
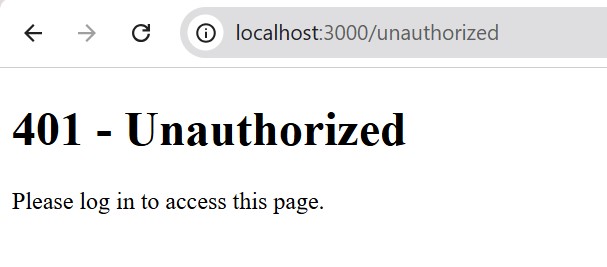
Example 2
You can invoke unauthorized in Server Actions to ensure only authenticated users can perform specific mutations. The code below shows how to perform a mutation that requires authentication.
'use server' import { verifySession } from '@/app/lib/dal' import { unauthorized } from 'next/navigation' import db from '@/app/lib/db' export async function updateProfile(data: FormData) { const session = await verifySession() // If the user is not authenticated, return a 401 if (!session) { unauthorized() } }