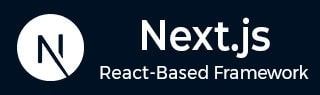
- Next.js - Home
- Next.js - Overview
- Next.js - Project Setup
- Next.js - Folder Structure
- Next.js - App Router
- Next.js - Page Router
- Next.js Features
- Next.js - Pages
- Next.js - Data Fetching
- Next.js - ISR
- Next.js - Static File Serving
- Next.js - Pre-Rendering
- Next.js - Partial Pre Rendering
- Next.js - Server Side Rendering
- Next.js - Client Side Rendering
- Next.js Routing
- Next.js - Routing
- Next.js - Nested Routing
- Next.js - Dynamic Routing
- Next.js - Parallel Routing
- Next.js - Imperative Routing
- Next.js - Shallow Routing
- Next.js - Intercepting Routes
- Next.js - Redirecting Routes
- Next.js - Navigation and Linking
- Next.js Configuration
- Next.js - TypeScript
- Next.js - Environment Variables
- Next.js - File Conventions
- Next.js - ESLint
- Next.js API & Backend
- Next.js - API Routes
- Next.js - Dynamic API Routes
- Next.js - Route Handlers
- Next.js - API MiddleWares
- Next.js - Response Helpers
- Next.js API Reference
- Next.js - CLI Commands
- Next.js - Functions
- Next.js - Directives
- Next.js - Components
- Next.js - Image Component
- Next.js - Font Component
- Next.js - Head Component
- Next.js - Form Component
- Next.js - Link Component
- Next.js - Script Component
- Next.js Styling & SEO
- Next.js - CSS Support
- Next.js - Global CSS Support
- Next.js - Meta Data
- Next.js Advanced Topics
- Next.js - Error Handling
- Next.js - Server Actions
- Next.js - Fast Refresh
- Next.js - Internationalization
- Next.js - Authentication
- Next.js - Session Management
- Next.js - Authorization
- Next.js - Caching
- Next.js - Data Caching
- Next.js - Router Caching
- Next.js - Full Route Caching
- Next.js - Request Memoization
- Next.js Performance Optimization
- Next.js - Optimizations
- Next.js - Image Optimization
- Next.js - Lazy Loading
- Next.js - Font Optimization
- Next.js - Video Optimization
- Next.js - Script Optimization
- Next.js - Memory Optimization
- Next.js - Using OpenTelemetry
- Next.js - Package Bundling Optimization
- Next.js Testing
- Next.js - Testing
- Next.js - Testing with Jest
- Next.js - Testing with Cypress
- Next.js - Testing with Vitest
- Next.js - Testing with Playwright
- Next.js Debugging & Deployment
- Next.js - Debugging
- Next.js - Deployment
- Next.js Useful Resources
- Next.js - Interview Questions
- Next.js - Quick Guide
- Next.js - Useful Resources
- Next.js - Discussion
Next.js - unstable_cache() Function
The unstable_cache() Function
The Next.js unstable_cache()
function is used to cache the results of expensive operations, like database queries, and reuse them across multiple requests. It's useful for improving the performance of your application.
Syntax
The syntax for the unstable_cache()
function is as follows:
import { unstable_cache } from 'next/cache' const data = unstable_cache(fetchData, keyParts, options)()
Parameters
The unstable_cache()
function accepts three parameters:
- fetchData: This is an asynchronous function that fetches the data you want to cache. It must be a function that returns a Promise.
- keyParts: This is an extra array of keys that further adds identification to the cache. By default, unstable_cache already uses the arguments and the stringified version of your function as the cache key. It is optional in most cases; the only time you need to use it is when you use external variables without passing them as parameters.
- options: This is an object that controls how the cache behaves.
Return Value
The unstable_cache()
returns a function that when invoked, returns a Promise that resolves to the cached data. If the data is not in the cache, the provided function will be invoked, and its result will be cached and returned.
Key Points
- The unstable_cache() function will be deprecated when use cache reaches stability and support in Next.js.
- The unstable_cache() function uses Next.js' built-in Data Cache to persist the result across requests and deployments.
Example 1
The code below shows how to use the unstable_cache() function to cache the result of a database query. The cache key is generated based on the user ID, and the cache is invalidated after 60 seconds.
import { unstable_cache } from 'next/cache' export default async function Page({ params, }: { params: Promise }) { const userId = (await params).userId const getCachedUser = unstable_cache( async () => { return { id: userId } }, [userId], // add the user ID to the cache key { tags: ['users'], revalidate: 60, } ) }
Example 2
The code below shows how to use the unstable_cache() function to invalidate the cache when a user logs out. The cache key is generated based on the user ID.
import { unstable_cache } from 'next/cache' export default async function Page({ params, }: { params: Promise }) { const userId = (await params).userId const getCachedUser = unstable_cache( async () => { return { id: userId } }, [userId], // add the user ID to the cache key { tags: ['users'], revalidate: 60, } ) }